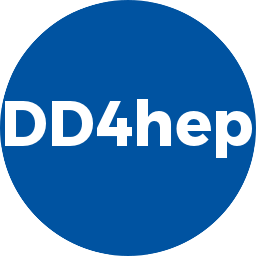 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSLINEARPOOL_H
14 #define DDCOND_CONDITIONSLINEARPOOL_H
44 template<
typename MAPPING,
typename BASE>
50 template <
typename R,
typename T>
size_t loop(R& result, T functor) {
51 size_t len = result.size();
53 return result.size() - len;
63 virtual size_t size() const final {
119 this->BASE::SetTitle(
"ConditionsLinearUpdatePool");
132 entries[o->iov].emplace_back(o);
145 unsigned int req_typ = req.iovType ? req.iovType->type : req.type;
146 const IOV::Key& req_key = req.key();
148 if (
key == e->hash ) {
149 const IOV* _iov = e->iov;
151 if ( req_typ == typ ) {
154 result.emplace_back(e);
157 result.emplace_back(e);
160 result.emplace_back(e);
172 #endif // DDCOND_CONDITIONSLINEARPOOL_H
194 template<
typename MAPPING,
typename BASE>
198 this->BASE::SetName(i ? i->
str().c_str() :
"Unknown IOV");
199 this->BASE::SetTitle(
"ConditionsLinearPool");
204 template<
typename MAPPING,
typename BASE>
207 detail::deletePtr(this->BASE::iov);
227 dd4hep::except(
"ConditionsLinearPool",
"++ Insufficient arguments: arg[0] = ConditionManager!");
235 dd4hep::except(
"ConditionsLinearPool",
"++ Insufficient arguments: arg[1] = IOV!");
239 #define _CR(fun,x,b,y) void* fun(dd4hep::Detector&, int argc, char** argv) \
240 { return new b<x<dd4hep::Condition::Object*>,y>(_mgr(argc,argv), _iov(argc,argv)); }
ConditionsOperation< KeyedSelect< C > > keyedSelect(Condition::key_type key, C &coll)
Helper to create functor to select keyed objects from a conditions pool.
Class implementing the conditions collection for a given IOV type.
std::vector< Condition > RangeConditions
AlignmentCondition::Object * cond
ConditionsOperation< KeyFind > keyFind(Condition::key_type key)
Helper to create functor to find conditions objects by hash key.
OperatorWrapper< oper_type > operatorWrapper(oper_type &oper)
Helper function to create a OperatorWrapper<T> object from the argument type.
Class implementing the conditions update pool for a given IOV type.
virtual size_t popEntries(UpdatePool::UpdateEntries &entries) final
Adopt all entries sorted by IOV. Entries will be removed from the pool.
ConditionsOperation< PoolRemove< P > > poolRemove(P &pool)
Helper to create functor to remove objects from a conditions pool.
virtual void select_range(Condition::key_type key, const IOV &req, RangeConditions &result) final
Select the conditions matching the DetElement and the conditions name.
std::string str() const
Create string representation of the IOV.
std::pair< Key_value_type, Key_value_type > Key
static void increment(T *)
Increment count according to type information.
virtual size_t select_all(ConditionsPool &result) final
Select the conditons, used also by the DetElement of the condition.
virtual void insert(RangeConditions &rc) final
Register a new condition to this pool. May overload for performance reasons.
Conditions selector functor. Default implementation selects everything evaluated.
virtual size_t select_all(const ConditionsSelect &result) final
Select the conditons, used also by the DetElement of the condition.
#define _CR(fun, x, b, y)
virtual Condition exists(Condition::key_type key) const final
Check if a condition exists in the pool.
Basic conditions manager implementation.
Main condition object handle.
Namespace for implementation details of the AIDA detector description toolkit.
virtual size_t size() const final
Total entry count.
Class describing the interval of validty.
ConditionsOperation< SequenceSelect< C > > sequenceSelect(C &coll)
Helper to create functor to select objects from a conditions pool into a sequential container.
virtual bool insert(Condition condition) final
Register a new condition to this pool.
Class implementing the conditions collection for a given IOV type.
static bool key_overlaps_lower_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the lower interval edge with IOV 'key'.
static void decrement(T *)
Decrement count according to type information.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
static bool key_overlaps_higher_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
virtual size_t select(Condition::key_type key, RangeConditions &result) final
Select the conditions matching the DetElement and the conditions name.
size_t loop(R &result, T functor)
Helper function to loop over the conditions container and apply a functor.
static bool key_is_contained(const Key &key, const Key &test)
Check if IOV 'test' is fully contained in IOV 'key'.
virtual void clear() final
Full cleanup of all managed conditions.
virtual ~ConditionsLinearPool()
Default destructor.
Key key() const
Get the local key of the IOV.
unsigned long long int key_type
Forward definition of the key type.
Manager class for condition handles.
unsigned int type
IOV buffer type: Must be a bitmap!
ConditionsLinearUpdatePool(ConditionsManager mgr, IOV *)
Default constructor.
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
std::map< const IOV *, ConditionEntries > UpdateEntries
Update container specification.
ConditionsOperation< PoolSelect< P > > poolSelect(P &pool)
Helper to create functor to insert objects into a conditions pool.
Interface for conditions pool optimized to host conditions updates.
virtual size_t select_all(RangeConditions &result) final
Select the conditons, used also by the DetElement of the condition.
unsigned int type
integer identifier used internally
ConditionsLinearPool(ConditionsManager mgr, IOV *iov)
Default constructor.
virtual ~ConditionsLinearUpdatePool()
Default destructor.