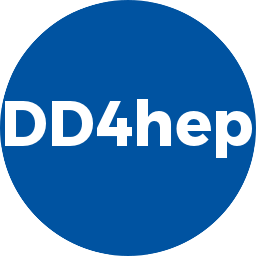 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
54 for(
const auto& c :
cond ) {
62 printout(WARNING,
"ConditionsContent",
63 "++ Condition %s already present in content. Not merged",
key.toString().c_str());
66 for(
const auto& d : deriv ) {
74 printout(WARNING,
"ConditionsContent",
75 "++ Dependency %s already present in content. Not merged",
key.toString().c_str());
83 detail::releasePtr((*i).second);
89 detail::releasePtr((*j).second);
96 std::pair<dd4hep::Condition::key_type, ConditionsLoadInfo*>
100 if ( ret.second )
return {
hash, 0 };
105 std::pair<dd4hep::Condition::key_type, ConditionsLoadInfo*>
120 std::pair<dd4hep::Condition::key_type, ConditionDependency*>
130 #if defined(DD4HEP_CONDITIONS_DEBUG)
132 const char* path = de.
isValid() ? de.
path().c_str() :
"(global)";
134 const char* path =
"";
137 except(
"DeConditionsRequests",
138 "++ Dependency already exists: %s [%08X] [%016llX]",
140 return std::pair<Condition::key_type, ConditionDependency*>(0,0);
144 std::pair<dd4hep::Condition::key_type, ConditionDependency*>
147 std::shared_ptr<ConditionUpdateCall> callback)
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
Dependencies m_derived
Container of derived conditions required by this content.
AlignmentCondition::Object * cond
Conditions m_conditions
Container of conditions required by this content.
Conditions & conditions()
Access to the real condition entries to be loaded.
std::size_t info(const std::string &src, const std::string &msg)
Condition::key_type hash
Hashed key representation.
ConditionKey target
Key to the condition to be updated.
bool isValid() const
Check the validity of the object held by the handle.
static void increment(T *)
Increment count according to type information.
void release()
Release object. May not be used any longer.
virtual ~ConditionsContent()
Default destructor.
DetElement detector
Reference to the target's detector element.
Key definition to optimize ans simplyfy the access to conditions entities.
Helper union to interprete conditions keys.
bool remove(Condition::key_type condition)
Remove a condition from the content.
virtual ~ConditionsLoadInfo()
Default destructor.
Namespace for implementation details of the AIDA detector description toolkit.
Conditions content object. Defines which conditions should be loaded by the ConditionsManager.
Condition dependency definition.
Dependencies & derived()
Access to the derived condition entries to be computed.
Handle class describing a detector element.
std::pair< Condition::key_type, ConditionsLoadInfo * > addLocationInfo(Condition::key_type hash, ConditionsLoadInfo *info)
Add a new conditions key. T must inherit from class ConditionsContent::Info.
Condition::key_type key() const
Access the dependency key.
void merge(const ConditionsContent &to_add)
Merge the content of "to_add" into the this content.
ConditionsContent()
Default constructor.
static void decrement(T *)
Decrement count according to type information.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
Base class for data loading information.
unsigned long long int key_type
Forward definition of the key type.
std::pair< Condition::key_type, ConditionDependency * > addDependency(ConditionDependency *dep)
Add a new shared conditions dependency.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
ConditionsLoadInfo()
Default constructor.
void clear()
Clear the conditions content definitions.
struct dd4hep::ConditionKey::KeyMaker::@2 values
std::pair< Condition::key_type, ConditionsLoadInfo * > insertKey(Condition::key_type hash)
Add a new conditions key representing a real (not derived) condition.
ConditionDependency * addRef()
Add use count to the object.
Condition::itemkey_type item_key