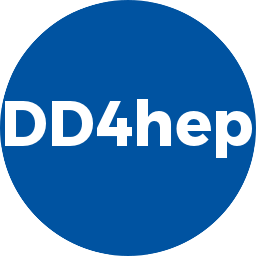 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DDCORE_VISVOLNAMEPROCESSOR_H
14 #define DD4HEP_DDCORE_VISVOLNAMEPROCESSOR_H
35 typedef std::map<std::string,std::regex>
Matches;
55 #endif // DD4HEP_DDCORE_VISVOLNAMEPROCESSOR_H
81 VisVolNameProcessor::VisVolNameProcessor(
Detector& desc)
82 : description(desc), name()
89 printout(ALWAYS,
name,
"++ %8ld vis-attrs '%s' applied.",
96 for(
const auto&
v : vals )
104 string vol_nam = vol.
name();
105 for (
const auto& match :
matches ) {
106 if ( std::regex_match(vol_nam, match.second) ) {
107 printout(ALWAYS,match.first,
"++ Set visattr %s to %s",
119 static void* create_object(
Detector& description,
int argc,
char** argv) {
121 vector<string> vol_names;
124 for (
int i=0; i<argc; ++i ) {
126 if ( ::strncmp(argv[i],
"-name",4) == 0 ) {
127 proc->
name = argv[++i];
128 vol_names.push_back(proc->
name);
129 if ( vis_name.empty() ) vis_name = proc->
name;
132 else if ( ::strncmp(argv[i],
"-match",4) == 0 ) {
133 vol_names.push_back(argv[++i]);
134 if ( vis_name.empty() ) vis_name = vol_names.back();
135 if ( proc->
name.empty() ) proc->
name = vol_names.back();
138 else if ( ::strncmp(argv[i],
"-vis",4) == 0 ) {
139 vis_name = argv[++i];
142 else if ( ::strncmp(argv[i],
"-show",4) == 0 ) {
147 "Usage: DD4hep_VisVolNameProcessor -arg [-arg] \n"
148 " -match <regex> Regular expression matching volume name \n"
149 " -show Print setup to output device (stdout) \n"
150 "\tArguments given: " << arguments(argc,argv) << endl << flush;
157 except(proc->
name,
"+++ Unknown visual attribute: %s",vis_name.c_str());
160 return (
void*)placement_proc;
Generic PlacedVolume processor.
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
DD4hep DetElement creator for the CMS geometry.
bool isValid() const
Check the validity of the object held by the handle.
const char * name() const
Access the object name (or "" if not supported by the object)
std::map< std::string, std::regex > Matches
Handle class holding a placed volume (also called physical volume)
VisVolNameProcessor(Detector &desc)
Initializing constructor.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
DetectorHelper: class to shortcut certain questions to the dd4hep detector description interface.
virtual const HandleMap & visAttributes() const =0
Accessor to the map of visualisation attributes.
T * ptr() const
Access to the held object.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Namespace for the AIDA detector description toolkit.
Volume volume() const
Logical volume of this placement.
The main interface to the dd4hep detector description package.
void set_match(const std::vector< std::string > &matches)
Set volume matches.
virtual ~VisVolNameProcessor()
Default destructor.
VisAttr visAttributes() const
Access the visualisation attributes.
virtual int operator()(PlacedVolume pv, int level)
Callback to output PlacedVolume information of an single Placement.
void _show()
Print properties.