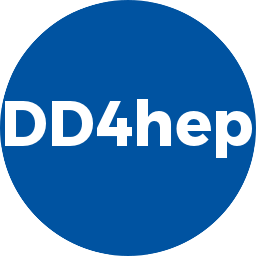 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <TEveManager.h>
25 #include <TEveWindow.h>
27 #include <TGLViewer.h>
32 static inline typename T::const_iterator find(
const T& cont,
const std::string& str) {
33 for(
typename T::const_iterator i=cont.begin(); i!=cont.end(); ++i)
34 if ( (*i).name == str )
return i;
40 : m_eve(eve), m_view(0), m_geoScene(0), m_eveScene(0), m_global(0), m_name(nam), m_showGlobal(false)
50 (*i).second->DestroyElements();
66 if ( evt ) evt->Repaint(kTRUE);
67 if ( geo ) geo->Repaint(kTRUE);
69 TGLViewer* glv =
viewer()->GetGLViewer();
70 glv->PostSceneBuildSetup(kTRUE);
71 glv->SetSmartRefresh(kFALSE);
73 glv->UseLightColorSet();
75 glv->UseDarkColorSet();
76 glv->RequestDraw(TGLRnrCtx::kLODHigh);
77 glv->SetSmartRefresh(kTRUE);
93 TEveScene* scene =
dynamic_cast<TEveScene*
>(el);
95 printf(
"ERROR: Adding a Scene [%s] to a list. This is BAD and causes crashes!\n",scene->GetName());
97 if ( el ) list->AddElement(el);
108 TEveScene* scene =
dynamic_cast<TEveScene*
>(el);
110 printf(
"ERROR: Adding a Scene [%s] to a list. This is BAD and causes crashes!\n",scene->GetName());
113 printout(INFO,
"View",
"ImportElement %s [%s] into list: %s",
115 list->AddElement(el);
121 std::pair<bool,TEveElement*>
125 TEveElement* elt = global.FindChild(de.
name());
126 return std::pair<bool,TEveElement*>(
true,elt);
130 std::pair<bool,TEveElement*>
139 printout(INFO,
"View",
"+++ Configure Geometry for view %s Config=%p.",
c_name(),
m_config);
148 for(TEveElementList::List_i i=l->BeginChildren(); i!=l->EndChildren(); ++i)
153 for(TEveElementList::List_i i=l->BeginChildren(); i!=l->EndChildren(); ++i)
160 DisplayConfiguration::Configurations::const_iterator ic;
166 std::string nam = cfg.
name;
167 if ( nam ==
"global" ) {
176 printout(INFO,
"View",
"+++ %s: add detector %s %s",
name().c_str(),nam.c_str(),ctx.
config.
use.c_str());
177 Color_t col = ctx.
calo3D->GetDataSliceColor(ctx.
slice);
179 legend_y += a->GetTextSize();
180 dets += nam +
"(Calo3D) ";
183 DetElement::Children::const_iterator i = c.find(nam);
184 if ( i != c.end() ) {
188 std::pair<bool,TEveElement*> e(
false,0);
193 if ( e.first && e.second ) {
196 dets += nam +
"(Geo) ";
200 printout(INFO,
"ViewConfiguration",
"+++ Configured geometry for view %s.",
c_name());
201 printout(INFO,
"ViewConfiguration",
"+++ Detectors:%s", dets.c_str());
206 printout(INFO,
"View",
"+++ %s: Import geometry topics.",
c_name());
208 printout(INFO,
"ViewConfiguration",
"+++ Add topic %s",(*i).second->GetName());
231 printout(INFO,
"View",
"+++ Import event data into view %s Config=%p.",
c_name(),
m_config);
239 for(TEveElementList::List_i i=l->BeginChildren(); i!=l->EndChildren(); ++i)
247 DisplayConfiguration::Configurations::const_iterator ic;
250 std::string nam = cfg.
name;
251 if ( nam ==
"global" ) {
260 TEveElement* child =
m_eve->
manager().GetEventScene()->FindChild(nam);
261 printout(INFO,
"View",
"+++ Add collection:%s data:%p scene:%p",nam.c_str(),child,
m_eveScene);
267 DetElement::Children::const_iterator i = c.find(nam);
273 TEveElement* child =
m_eve->
manager().GetEventScene()->FindChild(coll);
274 printout(INFO,
"View",
"+++ Add detector event %s collection:%s data:%p scene:%p",
302 return *((*i).second);
316 std::string nam =
m_name+
" - Event Data";
317 std::string tool =
m_name+
" - Scene holding projected event-data for the view.";
326 std::string nam =
m_name+
" - Geometry";
327 std::string tool =
m_name+
" - Scene holding projected geometry for the view.";
const Children & children() const
Access to the list of children.
virtual void ConfigureGeometry(const DisplayConfiguration::ViewConfig &config)
Configure a single geometry view.
The main class of the DDEve display.
const char * c_name() const
TEveScene * geoScene() const
Access to the Eve geometry scene.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
View(Display *eve, const std::string &name)
Initializing constructor.
static float DefaultTextSize()
Default text size.
Handle class to hold the information of a sensitive detector.
virtual std::pair< bool, TEveElement * > GetGlobalGeometry(DetElement de, const DisplayConfiguration::Config &cfg)
Access the global instance of the subdetector geometry.
virtual void ImportEvent(TEveElement *element)
Call to import event elements into the main event scene.
virtual void ConfigureGeometryFromGlobal()
Configure a single geometry view by default from the global geometry scene with all subdetectors.
virtual TEveElementList & GetGeoTopic(const std::string &name)
Access/Create an geometry topic by name.
union dd4hep::DisplayConfiguration::Config::Values data
virtual View & Map(TEveWindow *slot)
Map the view view to the slot.
Configurations subdetectors
bool isValid() const
Check the validity of the object held by the handle.
static void increment(T *)
Increment count according to type information.
Local implementation with overrides of the TEveElementList.
virtual std::pair< bool, TEveElement * > CreateGeometry(DetElement de, const DisplayConfiguration::Config &cfg)
Create a new instance of the geometry of a sub-detector.
const char * name() const
Access the object name (or "" if not supported by the object)
virtual ~View()
Default destructor.
virtual View & Build(TEveWindow *slot)
Build the view view and map it to the given slot.
virtual void ConfigureGeometryFromInfo()
Configure a view with geo info. Used configuration if present.
const ViewConfig * GetViewConfiguration(const std::string &name) const
Access a data filter by name. Data filters are used to customize views.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
TEveScene * eveScene() const
Access to the Eve event scene.
const DisplayConfiguration::ViewConfig * m_config
virtual TEveElementList & GetGeoTopic(const std::string &name)
Access/Create an geometry topic by name.
virtual TEveElement * ImportEventElement(TEveElement *element, TEveElementList *list)
Call an element to a event element list.
const std::string & name() const
Access to the view name/title.
Handle class describing a detector element.
const char * GetName(T *p)
CalodataContext & GetCaloHistogram(const std::string &name)
Access to calo data histograms by name as defined in the configuration.
virtual TEveElementList * AddToGlobalItems(const std::string &nam)
Add the view to the global list of eve objects.
View TEveWindowSlot * slot
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
static void decrement(T *)
Decrement count according to type information.
virtual TEveElement * ImportGeoElement(TEveElement *element, TEveElementList *list)
Call an element to a geometry element list.
virtual View & CreateGeoScene()
Create the geometry scene.
virtual View & CreateEventScene()
Create the event scene.
Detector & detectorDescription() const
Access to geometry hub.
static float DefaultMargin()
Default margin for placement in bottom left corner.
virtual TEveElement * ImportGeoTopic(TEveElement *element, TEveElementList *list)
Call an element to a geometry element list.
DisplayConfiguration::Config config
virtual void ImportEventTopics()
Import event typics after creation.
virtual void ConfigureEventFromInfo()
Configure a view with event info. Used configuration if present.
virtual void ConfigureEventFromGlobal()
Configure an event view by default from the global event scene.
TEveScene * m_geoScene
Reference to the geometry scene.
TEveViewer * viewer() const
Access to the Eve viewer.
Container with full display configuration.
std::pair< bool, TEveElement * > LoadDetElement(DetElement element, int levels, TEveElement *parent)
virtual void ImportGeoTopics(const std::string &title)
Call to import geometry topics. If title is empty, do not add to global item list.
std::map< std::string, DetElement > Children
Class to add annotations to eve viewers.
virtual View & CreateScenes()
Create the geometry and the event scene.
std::string m_name
The name of the view.
TEveElementList * m_global
Reference to the global item (if added.
TEveViewer * m_view
Reference to the view.
Namespace for the AIDA detector description toolkit.
virtual void Initialize()
Initialize the view port.
Readout readout() const
Access readout structure of the sensitive detector.
class View View.h DDEve/View.h
Calorimeter data context for the DDEve event display.
virtual void UnregisterEvents(View *view)
Unregister from the main event scene.
TEveScene * m_eveScene
Reference to the event scene.
TEveManager & manager() const
Access to the EVE manager.
virtual void ImportGeo(const std::string &topic, TEveElement *element)
Call to import geometry elements into topics.
virtual void ConfigureEvent(const DisplayConfiguration::ViewConfig &config)
Configure a single event scene view.