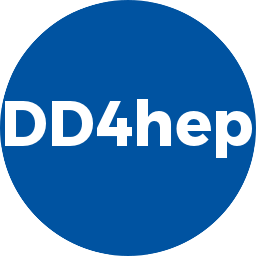 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <DD4hep/detail/Handle.inl>
35 const std::string& full_name,
41 std::unique_ptr<Object> obj(
new Object(full_name.c_str(), model, finish, type, value));
47 return access()->GetProperty(nam);
52 return access()->GetProperty(nam.c_str());
61 std::unique_ptr<Object> obj(
new Object(nam.c_str(), surf->GetName(), surf.
ptr(), vol.
ptr()));
65 except(
"SkinSurface",
"++ Cannot create SkinSurface %s without valid optical surface!",nam.c_str());
67 except(
"SkinSurface",
"++ Cannot create SkinSurface %s without valid volume!",nam.c_str());
70 "++ Cannot create SkinSurface %s which is not connected to a valid detector element!",nam.c_str());
75 return (TGeoOpticalSurface*)(
access()->GetSurface());
92 return access()->GetVolume();
98 const std::string& nam,
106 std::unique_ptr<Object> obj(
new Object(nam.c_str(), surf->GetName(), surf.
ptr(), lft.
ptr(), rht.
ptr()));
110 except(
"BorderSurface",
"++ Cannot create BorderSurface %s without valid optical surface!",nam.c_str());
112 except(
"BorderSurface",
"++ Cannot create BorderSurface %s without valid placements!",nam.c_str());
114 except(
"BorderSurface",
115 "++ Cannot create BorderSurface %s which is not connected to a valid detector element!",nam.c_str());
120 return (TGeoOpticalSurface*)(
access()->GetSurface());
137 return (TGeoNode*)
access()->GetNode1();
142 return access()->GetNode2();
Property property(const char *name) const
Access to tabular properties of the optical surface.
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
void addSkinSurface(DetElement de, SkinSurface surf) const
Add skin surface to manager.
PlacedVolume right() const
Access the right node of the border surface.
Handle class holding a placed volume (also called physical volume)
TGeoOpticalSurface Object
bool isValid() const
Check the validity of the object held by the handle.
Volume volume() const
Access the node of the skin surface.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
Object::ESurfaceFinish EFinish
const TGDMLMatrix * Property
BorderSurface()=default
Default constructor.
virtual OpticalSurfaceManager surfaceManager() const =0
Access the optical surface manager.
OpticalSurface surface() const
Access surface data.
TGeoOpticalSurface * m_element
Single and only data member: Reference to the actual element.
void addBorderSurface(DetElement de, BorderSurface surf) const
Add border surface to manager.
Property property(const char *name) const
Access to tabular properties of the optical surface.
OpticalSurface surface() const
Access surface data.
const TGDMLMatrix * Property
TGeoOpticalSurface * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
PlacedVolume left() const
Access the left node of the border surface.
DD4HEP_INSTANTIATE_HANDLE(TGeoSkinSurface)
Object::ESurfaceModel EModel
The main interface to the dd4hep detector description package.
SkinSurface()=default
Default constructor.
Property property(const char *name) const
Access to tabular properties of the surface.
Object::ESurfaceType EType
Class to support the handling of optical surfaces.
OpticalSurface()=default
Default constructor.