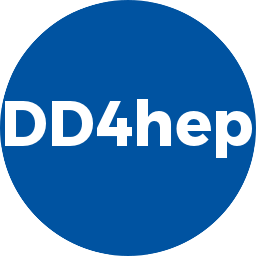 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
35 std::string n = de.
path() +
'#' + nam;
36 TGeoSkinSurface* surf = o->
detector.
manager().GetSkinSurface(n.c_str());
37 if ( surf )
return surf;
43 "++ Cannot access SkinSurface %s without valid detector element!",nam.c_str());
56 std::string n = de.
path() +
'#' + nam;
57 TGeoBorderSurface* surf = o->
detector.
manager().GetBorderSurface(n.c_str());
58 if ( surf )
return surf;
63 except(
"BorderSurface",
64 "++ Cannot access BorderSurface %s without valid detector element!",nam.c_str());
77 std::string n = de.
path() +
'#' + nam;
78 TGeoOpticalSurface* surf = o->
detector.
manager().GetOpticalSurface(n.c_str());
79 if ( surf )
return surf;
84 except(
"OpticalSurface",
85 "++ Cannot access OpticalSurface %s without valid detector element!",nam.c_str());
96 if (
access()->skinSurfaces.emplace(std::make_pair(de,surf->GetName()), surf).second )
98 except(
"OpticalSurfaceManager",
"++ Skin surface %s already present for DE:%s.",
99 surf->GetName(), de.
name());
104 if (
access()->borderSurfaces.emplace(std::make_pair(de,surf->GetName()), surf).second )
106 except(
"OpticalSurfaceManager",
"++ Border surface %s already present for DE:%s.",
107 surf->GetName(), de.
name());
112 if (
access()->opticalSurfaces.emplace(surf->GetName(), surf).second )
114 except(
"OpticalSurfaceManager",
"++ Optical surface %s already present.",
124 extension->opticalSurfaces.insert(optical);
129 std::string n = skin.first.first.path() +
'#' + skin.first.second;
130 skin.second->SetName(n.c_str());
132 extension->skinSurfaces.insert(skin);
137 std::string n = border.first.first.path() +
'#' + border.first.second;
138 border.second->SetName(n.c_str());
140 extension->borderSurfaces.insert(border);
144 if ( extension->opticalSurfaces.empty() &&
145 extension->borderSurfaces.empty() &&
146 extension->skinSurfaces.empty() ) {
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
OpticalSurface opticalSurface(const std::string &full_name) const
Access optical surface data by its full name.
This structure describes the internal data of the volume manager object.
Detector & detector
Reference to the main detector description object.
void addSkinSurface(DetElement de, SkinSurface surf) const
Add skin surface to manager.
bool isValid() const
Check the validity of the object held by the handle.
void addOpticalSurface(OpticalSurface surf) const
Add optical surface data to manager.
static OpticalSurfaceManager getOpticalSurfaceManager(Detector &description)
static accessor calling DD4hepOpticalSurfaceManagerPlugin if necessary
const char * name() const
Access the object name (or "" if not supported by the object)
Handle class describing a detector element.
std::map< LocalKey, SkinSurface > skinSurfaces
Implementation class for the object extension mechanism.
void registerSurfaces(DetElement subdetector)
Register the temporary surface objects with the TGeoManager.
Class to support the handling of optical surfaces.
virtual OpticalSurfaceManager surfaceManager() const =0
Access the optical surface manager.
void addBorderSurface(DetElement de, BorderSurface surf) const
Add border surface to manager.
BorderSurface borderSurface(const std::string &full_name) const
Access border surface by its full name.
detail::OpticalSurfaceManagerObject Object
detail::OpticalSurfaceManagerObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
std::map< LocalKey, BorderSurface > borderSurfaces
std::pair< DetElement, std::string > LocalKey
void * addExtension(ExtensionEntry *entry) const
Add an extension object to the detector element.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
Class to support the handling of optical surfaces.
std::map< std::string, OpticalSurface > opticalSurfaces
Class to support the handling of optical surfaces.
SkinSurface skinSurface(const std::string &full_name) const
Access skin surface by its full name.
Class to support the handling of optical surfaces.