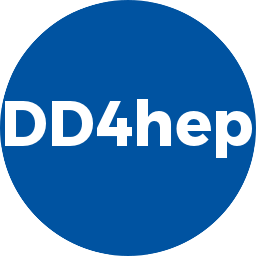 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_OPTICALSURFACES_H
14 #define DD4HEP_OPTICALSURFACES_H
21 #include <TGeoOpticalSurface.h>
48 typedef Object::ESurfaceModel
EModel;
50 typedef Object::ESurfaceType
EType;
67 const std::string&
name,
68 EModel model = Model::kMglisur,
69 EFinish finish = Finish::kFpolished,
70 EType type = Type::kTdielectric_dielectric,
84 return Object::StringToType(type.c_str());
88 return Object::TypeToString(type);
91 return Object::StringToModel(model.c_str());
94 return Object::ModelToString(model);
97 return Object::StringToFinish(finish.c_str());
100 return Object::FinishToString(finish);
126 template <
typename Q>
131 const std::string& nam,
170 template <
typename Q>
175 const std::string& nam,
194 #endif // DD4HEP_OPTICALSURFACES_H
Property property(const char *name) const
Access to tabular properties of the optical surface.
BorderSurface(Object *obj)
Constructor taking object pointer.
static EType stringToType(const std::string &type)
Convenience function forwarding to TGeoOpticalSurface.
const TGDMLMatrix * Property
OpticalSurface & operator=(const OpticalSurface &m)=default
Assignment operator.
PlacedVolume right() const
Access the right node of the border surface.
Handle class holding a placed volume (also called physical volume)
TGeoOpticalSurface Object
static std::string typeToString(EType type)
Convenience function forwarding to TGeoOpticalSurface.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
OpticalSurface(Object *obj)
Constructor taking object pointer.
const char * name() const
Access the object name (or "" if not supported by the object)
BorderSurface(const BorderSurface &e)=default
Copy constructor.
OpticalSurface(const OpticalSurface &e)=default
Copy constructor.
Volume volume() const
Access the node of the skin surface.
SkinSurface(const Handle< Object > &e)
Constructor from same-type handle.
Handle class describing a detector element.
BorderSurface & operator=(const BorderSurface &m)=default
Assignment operator.
Handle class holding a placed volume (also called physical volume)
Object::ESurfaceFinish EFinish
BorderSurface(const Handle< Object > &e)
Constructor from same-type handle.
SkinSurface(const SkinSurface &e)=default
Copy constructor.
const TGDMLMatrix * Property
Class to support the handling of optical surfaces.
SkinSurface(Object *obj)
Constructor taking object pointer.
BorderSurface()=default
Default constructor.
SkinSurface(const Handle< Q > &e)
Constructor from arbitrary handle.
OpticalSurface surface() const
Access surface data.
OpticalSurface(const Handle< Object > &e)
Constructor from same-type handle.
Property property(const char *name) const
Access to tabular properties of the optical surface.
OpticalSurface surface() const
Access surface data.
const TGDMLMatrix * Property
static EModel stringToModel(const std::string &model)
BorderSurface(const Handle< Q > &e)
Constructor from arbitrary handle.
Namespace for the AIDA detector description toolkit.
PlacedVolume left() const
Access the left node of the border surface.
Object::ESurfaceModel EModel
static std::string modelToString(EModel model)
The main interface to the dd4hep detector description package.
static EFinish stringToFinish(const std::string &finish)
SkinSurface()=default
Default constructor.
Property property(const char *name) const
Access to tabular properties of the surface.
Class to support the handling of optical surfaces.
Object::ESurfaceType EType
SkinSurface & operator=(const SkinSurface &m)=default
Assignment operator.
Class to support the handling of optical surfaces.
OpticalSurface()=default
Default constructor.
OpticalSurface(const Handle< Q > &e)
Constructor from arbitrary handle.
static std::string finishToString(EFinish finish)