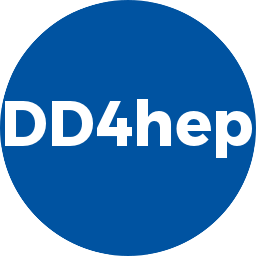 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
32 #include <HepMC3/ReaderFactory.h>
33 #include <HepMC3/Version.h>
42 for(
auto const& attr: genEvent.attributes()){
43 for(
auto const& inAttr: attr.second){
44 std::stringstream strstr;
47 if(attr.second.size() > 1 or inAttr.first != 0){
48 strstr <<
"_" << inAttr.first;
50 if(
auto int_attr = std::dynamic_pointer_cast<HepMC3::IntAttribute>(inAttr.second)) {
52 }
else if(
auto flt_attr = std::dynamic_pointer_cast<HepMC3::FloatAttribute>(inAttr.second)) {
54 }
else if(
auto dbl_attr = std::dynamic_pointer_cast<HepMC3::DoubleAttribute>(inAttr.second)) {
57 m_strValues[strstr.str()] = {inAttr.second->unparsed_string()};
62 if (
const auto& weights = genEvent.weights(); !weights.empty()) {
68 if (genEvent.run_info() && !genEvent.run_info()->weight_names().empty()) {
69 m_strValues[
"EventWeightNames"] = genEvent.weight_names();
75 for(
auto const& attr: runInfo.attributes()){
76 if(
auto int_attr = std::dynamic_pointer_cast<HepMC3::IntAttribute>(attr.second)) {
78 }
else if(
auto flt_attr = std::dynamic_pointer_cast<HepMC3::FloatAttribute>(attr.second)) {
80 }
else if(
auto dbl_attr = std::dynamic_pointer_cast<HepMC3::DoubleAttribute>(attr.second)) {
83 m_strValues[attr.first] = {attr.second->unparsed_string()};
125 HEPMC3FileReader::HEPMC3FileReader(
const std::string& nam)
127 : HEPMC3EventReader(nam)
129 printout(INFO,
"HEPMC3FileReader",
"Created file reader. Try to open input %s", nam.c_str());
130 m_reader = HepMC3::deduce_reader(nam);
131 #if HEPMC3_VERSION_CODE >= 3002006
133 HepMC3::GenEvent dummy;
136 auto runInfo =
m_reader->run_info();
140 m_reader = HepMC3::deduce_reader(nam);
142 m_reader->set_run_info(std::move(runInfo));
150 parameters->ingestParameters(*(
m_reader->run_info()));
153 printout(ERROR,
"HEPMC3FileReader::registerRunParameters",
"Failed to register run parameters: %s", e.what());
160 printout(INFO,
"HEPMC3FileReader::moveToEvent",
"Skipping the first %d events ", event_number);
162 printout(INFO,
"HEPMC3FileReader::moveToEvent",
"Event number before skipping: %d",
m_currEvent );
163 HepMC3::GenEvent genEvent;
164 auto status_OK =
m_reader->skip(event_number);
169 printout(INFO,
"HEPMC3FileReader::moveToEvent",
"Event number after skipping: %d",
m_currEvent );
177 auto status_OK =
m_reader->read_event(genEvent);
182 if (genEvent.particles().size()) {
183 printout(INFO,
"HEPMC3FileReader",
"Read event from file");
Base class to read hepmc3 event files.
Geant4Context * context() const
Get the context (from the input action)
std::shared_ptr< HepMC3::Reader > m_reader
Reference to reader object.
EventReaderStatus
Status codes of the event reader object. Anything with NOT low-bit set is an error.
void exception(const std::string &src, const std::string &msg)
std::map< std::string, std::vector< std::string > > m_strValues
Geant4Run & run() const
Access the geant4 run – valid only between BeginRun() and EndRun()!
void _getParameterValue(std::map< std::string, std::string > ¶meters, std::string const ¶meterName, T ¶meter, T defaultValue)
transform the string parameter value into the type of parameter
void setEventNumber(int eventNumber)
#define DECLARE_GEANT4_EVENT_READER_NS(name_space, name)
Plugin defintion to create event reader objects.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
int m_currEvent
Current event number.
Extension to pass input run data to output run data.
virtual EventReaderStatus readGenEvent(int event_number, HepMC3::GenEvent &genEvent)
Read an event and fill a vector of MCParticles.
virtual ~HEPMC3FileReader()=default
Default destructor.
void * addExtension(unsigned long long int k, ExtensionEntry *e)
Add an extension object to the detector element.
void ingestParameters(T const &source)
Copy the parameters from source.
std::string m_flow2
name of the GenEvent Attribute storing the color flow2
std::map< std::string, std::vector< int > > m_intValues
bool m_directAccess
Flag if direct event access is supported. To be explicitly set by subclass constructors.
std::map< std::string, std::vector< double > > m_dblValues
virtual EventReaderStatus moveToEvent(int event_number)
skip to event with event_number
Basic geant4 event reader class. This interface/base-class must be implemented by concrete readers.
virtual EventReaderStatus setParameters(std::map< std::string, std::string > ¶meters)
Set the parameters for the class.
std::map< std::string, std::vector< float > > m_fltValues
virtual void registerRunParameters()
register the run parameters into an extension for the run context
std::string m_flow1
name of the GenEvent Attribute storing the color flow1
void * addExtension(unsigned long long int k, ExtensionEntry *e)
Add an extension object to the detector element.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Event extension to pass input event data to output event.
Namespace for the AIDA detector description toolkit.
HEPMC3FileReader(const std::string &nam)
Initializing constructor.
void ingestParameters(T const &source)
Copy the parameters from source.
Generic context to extend user, run and event information.