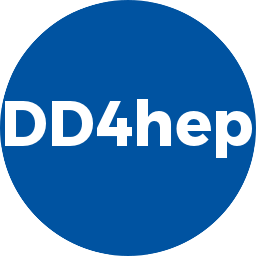 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
12 #ifndef DDG4_RUNPARAMETERS_H
13 #define DDG4_RUNPARAMETERS_H
45 #endif // DDG4_RUNPARAMETERS_H
Extension to pass input data to output data.
Extension to pass input run data to output run data.
void setRunNumber(int runNumber)
Set the Run parameters.
void extractParameters(T &destination)
Put parameters into destination.
int runNumber() const
Get the run number.
Namespace for the AIDA detector description toolkit.
void ingestParameters(T const &source)
Copy the parameters from source.