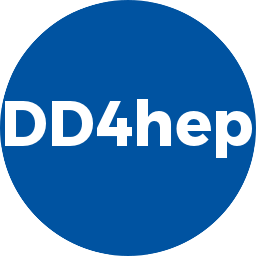 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
15 namespace DDSegmentation {
21 _description =
"Phi-eta segmentation in the global coordinates";
36 _description =
"Phi-eta segmentation in the global coordinates";
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
std::string m_phiID
the field name used for phi
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID get(CellID bitfield, size_t idx) const
int m_phiBins
the number of bins in phi
double m_offsetEta
the coordinate offset in eta
double m_offsetPhi
the coordinate offset in phi
virtual Vector3D position(const CellID &aCellID) const
GridPhiEta(const std::string &aCellEncoding)
default constructor using an arbitrary type
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
double phiFromXYZ(const Vector3D &position)
calculates the azimuthal angle phi from Cartesian coordinates
std::string m_etaID
the field name used for eta
void set(CellID &bitfield, size_t idx, FieldID value) const
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
double eta(const CellID &aCellID) const
double m_gridSizeEta
the grid size in eta
std::string _description
The description of the segmentation.
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
Vector3D positionFromREtaPhi(double ar, double aeta, double aphi)
calculates the Cartesian position from spherical coordinates (r, phi, eta)
Namespace for the AIDA detector description toolkit.
std::string _type
The segmentation type.
double phi(const CellID &aCellID) const
Base class for all segmentations.
double etaFromXYZ(const Vector3D &aposition)
calculates the pseudorapidity from Cartesian coordinates
virtual CellID cellID(const Vector3D &aLocalPosition, const Vector3D &aGlobalPosition, const VolumeID &aVolumeID) const