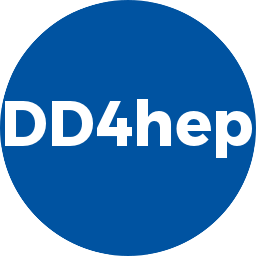 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #ifndef DDSEGMENTATION_SEGMENTATIONUTIL_H
19 #define DDSEGMENTATION_SEGMENTATIONUTIL_H
26 namespace DDSegmentation {
44 return std::sqrt(position.
X * position.
X + position.
Y * position.
Y + position.
Z * position.
Z);
49 return std::sqrt(position.
X * position.
X + position.
Y * position.
Y);
64 return std::atan2(position.
Y, position.
X);
71 if (cosTheta*cosTheta < 1)
return -0.5* std::log((1.0-cosTheta)/(1.0+cosTheta));
72 if (aposition.
Z == 0)
return 0;
74 if (aposition.
Z > 0)
return 10e10;
84 return Vector3D(r * std::cos(phi), r * std::sin(phi), z);
89 return std::sqrt(r * r + z * z);
93 inline double xFromRPhiZ(
double r,
double phi,
double ) {
94 return r * std::cos(phi);
98 inline double yFromRPhiZ(
double r,
double phi,
double ) {
99 return r * std::sin(phi);
104 return r * std::atan(z / r);
113 return Vector3D(r * std::cos(phi), r * std::sin(phi), r * std::tan(theta));
118 double r = mag * sin(theta);
119 return Vector3D(r * std::cos(phi), r * std::sin(phi), mag * std::cos(theta));
123 return Vector3D(ar * std::cos(aphi), ar * std::sin(aphi), ar * std::sinh(aeta));
131 #endif // DDSEGMENTATION_SEGMENTATIONUTIL_H
Vector3D positionFromRThetaPhi(double r, double theta, double phi)
Conversions from spherical to Cartesian coordinates ///.
Vector3D positionFromRPhiZ(double r, double phi, double z)
Conversions from cylindrical to Cartesian coordinates ///.
Simple container for a physics vector.
double radiusFromXYZ(const Vector3D &position)
calculates the radius in the xy-plane from Cartesian coordinates
double cosThetaFromXYZ(const Vector3D &position)
Calculates cosine of the polar angle theat from Cartesian coodinates.
double thetaFromXYZ(const Vector3D &position)
calculates the polar angle theta from Cartesian coordinates
double magFromXYZ(const Vector3D &position)
Conversions from Cartesian to cylindrical/spherical coordinates ///.
double yFromRPhiZ(double r, double phi, double)
calculates y from cylindrical coordinates
Vector3D positionFromMagThetaPhi(double mag, double theta, double phi)
calculates the Cartesian position from spherical coordinates
double phiFromXYZ(const Vector3D &position)
calculates the azimuthal angle phi from Cartesian coordinates
double thetaFromRPhiZ(double r, double, double z)
calculates the polar angle theta from cylindrical coordinates
double xFromRPhiZ(double r, double phi, double)
calculates x from cylindrical coordinates
Vector3D positionFromREtaPhi(double ar, double aeta, double aphi)
calculates the Cartesian position from spherical coordinates (r, phi, eta)
double magFromRPhiZ(double r, double, double z)
calculates the radius in xyz from cylindrical coordinates
Namespace for the AIDA detector description toolkit.
double etaFromXYZ(const Vector3D &aposition)
calculates the pseudorapidity from Cartesian coordinates