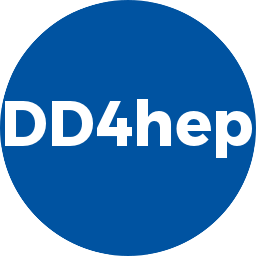 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <G4Version.hh>
22 #include <G4VisExecutive.hh>
23 #include <G4UImanager.hh>
24 #include <G4UIsession.hh>
25 #include <G4VisExecutive.hh>
26 #include <G4UIExecutive.hh>
27 #include <G4RunManager.hh>
36 std::string make_cmd(
const std::string& cmd) {
37 return std::string(
"/control/execute "+cmd);
71 G4UImanager* mgr = G4UImanager::GetUIpointer();
78 info(
"++ Executing configure command: %s",c.c_str());
79 G4int ret = mgr->ApplyCommand(c.c_str());
81 except(
"Failed to execute command: %s",c.c_str());
89 G4UImanager* mgr = G4UImanager::GetUIpointer();
92 info(
"++ Executing initialization command: %s",c.c_str());
93 G4int ret = mgr->ApplyCommand(c.c_str());
95 except(
"Failed to execute command: %s",c.c_str());
103 G4UImanager* mgr = G4UImanager::GetUIpointer();
106 info(
"++ Executing finalization command: %s",c.c_str());
107 G4int ret = mgr->ApplyCommand(c.c_str());
109 except(
"Failed to execute command: %s",c.c_str());
117 G4UImanager* mgr = G4UImanager::GetUIpointer();
119 info(
"++ Executing G4 command: %s",command.c_str());
120 G4int ret = mgr->ApplyCommand(command.c_str());
124 except(
"Failed to execute command: %s",command.c_str());
126 except(
"No UI reference present. Too early to interact with Geant4!");
144 info(
"++ End of processing requested.");
152 info(
"+++ Starting G4VisExecutive ....");
153 G4VisManager* vis =
new G4VisExecutive();
160 G4UIExecutive* ui = 0;
161 const char* args[] = {
"DDG4",
"",
""};
162 info(
"+++ Starting G4UIExecutive '%s' of type %s....", args[0],
m_sessionType.c_str());
163 #if (G4VERSION_NUMBER >= 960)
164 ui =
new G4UIExecutive(1,(
char**)args,
m_sessionType.c_str());
166 ui =
new G4UIExecutive(1,(
char**)args );
183 G4UImanager* mgr = G4UImanager::GetUIpointer();
184 bool executed_statements =
false;
195 mgr->ApplyCommand(make_cmd(
m_visSetup).c_str());
200 mgr->ApplyCommand(make_cmd(
m_uiSetup).c_str());
201 executed_statements =
true;
205 info(
"++ Executing Macro file: %s",m.c_str());
206 mgr->ApplyCommand(make_cmd(m.c_str()));
207 executed_statements =
true;
211 info(
"++ Executing pre-run statement: %s",c.c_str());
212 G4int ret = mgr->ApplyCommand(c.c_str());
214 except(
"Failed to execute command: %s",c.c_str());
216 executed_statements =
true;
220 m_ui->SessionStart();
223 info(
"++ Executing post-run statement: %s",c.c_str());
224 G4int ret = mgr->ApplyCommand(c.c_str());
226 except(
"Failed to execute command: %s",c.c_str());
228 executed_statements =
true;
233 warning(
"++ No UI manager found. Exit.");
236 else if ( executed_statements ) {
239 info(
"++ Executing post-run statement: %s",c.c_str());
240 G4int ret = mgr->ApplyCommand(c.c_str());
242 except(
"Failed to execute command: %s",c.c_str());
250 if(numEvent < 0) numEvent = std::numeric_limits<int>::max();
251 info(
"++ Start run with %d events.",numEvent);
256 info(
"++ End of file reached, ending run...");
263 detail::deletePtr(
m_vis);
264 detail::deletePtr(
m_ui);
void terminate()
Callback on terminate. Callback registered to the Geant4Kernel.
bool m_haveUI
Property: Flag to instantiate UI (default=true)
std::vector< std::string > m_initializeCommands
Property: List of commands to be executed when the Geant4Kernel gets initialized.
virtual ~Geant4UIManager()
Default destructor.
G4VisManager * m_vis
Reference to Geant4 visualtion manager.
void start()
Start manager & session.
std::vector< std::string > m_macros
Property: Array of commands to be chained.
G4UIExecutive * m_ui
Reference to Geant4 UI manager.
Property & property(const std::string &name)
Access single property.
void forceExit()
Force exiting this process without calling atexit handlers.
std::vector< std::string > m_terminateCommands
Property: List of commands to be executed when the Geant4Kernel gets terminated.
std::string m_visSetup
Property: Name of the visualization macro file.
void warning(const char *fmt,...) const
Support of warning messages.
void initialize()
Initialize the object. Callback registered to the Geant4Kernel.
void register_initialize(const std::function< void()> &callback)
Register initialize callback. Signature: (function)()
G4UIExecutive * startUI()
Start UI.
bool m_haveVis
Property: Flag to instantiate Vis manager (default=false, unless m_visSetup set)
void stop()
Stop and release resources.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
void register_terminate(const std::function< void()> &callback)
Register terminate callback. Signature: (function)()
std::string m_uiSetup
Property: Name of the UI macro file.
Definition of the generic callback structure for member functions.
void installCommandMessenger()
Install command control messenger to write GDML file from command prompt.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
void regularExit()
Regularly exiting this process without calling atexit handlers.
Default base class for all Geant 4 actions and derivates thereof.
void applyCommand(const std::string &command)
Apply single command.
void configure()
Configure the object. Callback registered to the Geant4Kernel.
void register_configure(const std::function< void()> &callback)
Register configure callback. Signature: (function)()
TYPE value() const
Retrieve value.
Geant4UIMessenger * m_control
Control directory of this action.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
Helper class to indicate the end of the input file.
G4VisManager * startVis()
Start visualization.
virtual void operator()(void *param)
Run UI.
void addCall(const std::string &name, const std::string &description, const Callback &cb, size_t npar=0)
Add a new callback structure.
std::string m_sessionType
Name of the default session type (="cmd")
std::vector< std::string > m_preRunCommands
Property: List of commands to be executed BEFORE running.
std::vector< std::string > m_configureCommands
Property: List of commands to be executed when the Geant4Kernel gets configured.
virtual void enableUI()
Enable and install UI messenger.
G4RunManager & runManager()
Access to the Geant4 run manager.
std::vector< std::string > m_postRunCommands
Property: List of commands to be executed AFTER running.
std::string m_prompt
Property: New prompt if the user wants to change it. (Default is do nothing)
Geant4UIManager(Geant4Context *context, const std::string &name)
Initializing constructor.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
virtual int terminate() override
Run the simulation: Terminate Geant4.