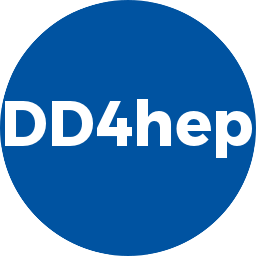 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
25 #include <CLHEP/Random/EngineFactory.h>
60 dd4hep::printout( dd4hep::INFO,
m_type,
"Get RunID: runID=%u",
m_runID );
69 unsigned int eventID = evt->GetEventID();
72 dd4hep::printout( dd4hep::INFO,
m_type,
73 "At beginEvent: eventID=%u, runID=%u initialSeed=%u, newSeed=%u" ,
78 if ( dd4hep::printLevel() <= dd4hep::DEBUG ) {
static Geant4Random * instance(bool throw_exception=true)
Access the main Geant4 random generator instance. Must be created before used!
CLHEP::HepRandomEngine * engine()
CLHEP random number engine (valid after initialization only)
void begin(const G4Run *)
begin-of-run callback
virtual ~Geant4EventSeed()
Default destructor.
void callAtBegin(Q *p, void(T::*f)(const G4Run *))
Register begin-of-run callback. Types Q and T must be polymorph!
static void increment(T *)
Increment count according to type information.
Plugin class to set the event seed for each event.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
Geant4EventSeed(Geant4Context *, const std::string &)
Standard constructor with initializing arguments.
virtual void setSeed(long seed)
Should initialise the status of the algorithm according to seed.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
unsigned int m_initialSeed
void callAtBegin(Q *p, void(T::*f)(const G4Event *))
Register begin-of-event callback.
virtual void showStatus() const
Should dump the current engine status on the screen.
static void decrement(T *)
Decrement count according to type information.
void beginEvent(const G4Event *)
begin-of-event callback
Mini interface to THE random generator of the application.
Geant4RunActionSequence & runAction() const
Access to the main run action sequence from the kernel object.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Concrete basic implementation of the Geant4 run action base class.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
Generic context to extend user, run and event information.