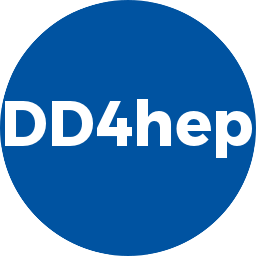 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
15 #ifndef DD4HEP_CONDITIONS_MULTICONDITONSLOADER_H
16 #define DD4HEP_CONDITIONS_MULTICONDITONSLOADER_H
29 class ConditionsHandler;
38 typedef std::map<std::string, ConditionsDataLoader*>
Loaders;
39 typedef std::map<std::string, ConditionsDataLoader*>
OpenSources;
53 const IOV& req_validity,
57 const IOV& req_validity,
66 except(
"ConditionsLoader",
"+++ update: Invalid call!");
100 const char* name = argc>0 ? argv[0] :
"MULTILoader";
119 const IOV& req_validity)
123 size_t idx = nam.find(
':');
124 if ( idx == std::string::npos ) {
125 except(
"ConditionsMultiLoader",
"Invalid data source specification: "+nam);
127 std::string ident = nam.substr(0,idx);
128 Loaders::iterator ild =
m_loaders.find(ident);
131 std::string typ =
"DD4hep_Conditions_"+ident+
"_Loader";
132 std::string fac = ident+
"_ConditionsDataLoader";
133 const void* argv[] = {fac.c_str(),
m_mgr.
ptr(), 0};
134 loader = createPlugin<ConditionsDataLoader>(typ,
m_detector,2,argv);
136 except(
"ConditionsMultiLoader",
137 "Failed to create conditions loader of type: "+typ+
" to read:"+nam);
141 loader = (*ild).second;
143 loader->
addSource(nam.substr(idx+1),req_validity);
148 return (*iop).second;
151 size_t ConditionsMultiLoader::load_range(key_type
key,
153 const IOV& req_validity,
156 size_t len = conditions.size();
159 const IOV& iov = (*i).second;
160 if ( iov.
type == req_validity.
type ) {
162 const std::string& nam = (*i).first;
164 loader->load_range(
key, req_validity, conditions);
168 return conditions.size() - len;
172 size_t ConditionsMultiLoader::load_single(key_type
key,
173 const IOV& req_validity,
176 size_t len = conditions.size();
179 const IOV& iov = (*i).second;
180 if ( iov.type == req_validity.type ) {
182 const std::string& nam = (*i).first;
184 loader->load_single(
key, req_validity, conditions);
188 return conditions.size() - len;
std::map< std::string, ConditionsDataLoader * > Loaders
Condition::key_type key_type
std::vector< Condition > RangeConditions
AlignmentCondition::Object * cond
static bool key_partially_contained(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
virtual ~ConditionsMultiLoader()
Default destructor.
ConditionsDataLoader * load_source(const std::string &nam, const IOV &req_validity)
Sources m_sources
Property: input data source definitions.
std::map< std::string, ConditionsDataLoader * > OpenSources
void addSource(const std::string &source)
Add data source definition to loader.
Basic conditions manager implementation.
Namespace for implementation details of the AIDA detector description toolkit.
Class describing the interval of validty.
Implementation of a stack of conditions assembled before application.
ConditionsManager m_mgr
Reference to conditions manager used to queue update requests.
std::vector< std::pair< key_type, ConditionsLoadInfo * > > RequiredItems
Key keyData
IOV key (if second==first, discrete, otherwise range)
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
OpenSources m_openSources
Manager class for condition handles.
unsigned int type
IOV buffer type: Must be a bitmap!
virtual size_t load_many(const IOV &, RequiredItems &, LoadedItems &, IOV &)
Optimized update using conditions slice data.
Interface for a generic conditions loader.
T * ptr() const
Access to the held object.
ConditionsMultiLoader(Detector &description, ConditionsManager mgr, const std::string &nam)
Default constructor.
Detector & m_detector
Reference to main detector description object.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
std::map< key_type, Condition > LoadedItems