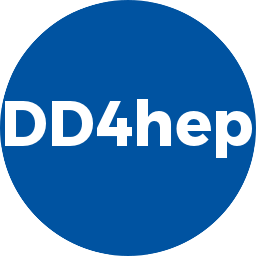 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
39 if ( res ==
data.end() ) {
40 if (
key == Keys::alignmentKey ) {
69 for(
const auto& i :
data )
77 dd4hep::except(
"AlignmentsNominalMap",
78 "Cannot scan conditions map for conditions of an invalid top level detector element!");
93 for(
auto i=
data.lower_bound(low); i !=
data.end() && (*i).first <= up; ++i) {
95 if ( low <= k.hash && up >= k.
hash ) {
96 processor((*i).second);
102 if ( align_key.
hash ) {
103 if ( lower <= Keys::alignmentKey && upper >= Keys::alignmentKey ) {
110 dd4hep::except(
"AlignmentsNominalMap",
111 "Cannot scan conditions map for conditions of an invalid detector element!");
Helper to run DetElement scans.
virtual void scan(const Condition::Processor &processor) const override
Interface to scan data content of the alignments mapping.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
virtual Condition get(DetElement detector, Condition::itemkey_type key) const override
Interface to access alignments by hash value. The detector element key and the item key make a unique...
bool isValid() const
Check the validity of the object held by the handle.
Alignment and Delta item key.
Key definition to optimize ans simplyfy the access to conditions entities.
Helper union to interprete conditions keys.
DetElement world
Reference to the top detector element.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
Handle class describing a detector element.
int scan(Q &p, DetElement start, int level=0, bool recursive=true) const
Detector element tree scanner using wrapped DetectorProcessor objects.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition) override
Insert a new entry to the map. The detector element key and the item key make a unique global alignme...
unsigned long long int key_type
Forward definition of the key type.
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
std::map< Condition::key_type, Condition > data
Potential cache of real conditions.
Abstract base for processing callbacks to conditions objects.
AlignmentsNominalMap(DetElement wrld)
Standard constructor.