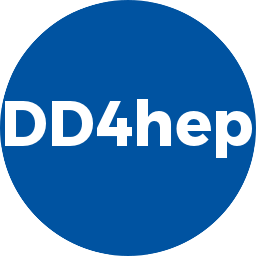 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 namespace DDSegmentation {
27 _type =
"PolarGridRPhi2";
28 _description =
"Polar RPhi segmentation in the local XY-plane";
43 _type =
"PolarGridRPhi2";
44 _description =
"Polar RPhi segmentation in the local XY-plane";
71 cellPosition.
X = R * cos(phi);
72 cellPosition.
Y = R * sin(phi);
80 double phi = atan2(localPosition.
Y,localPosition.
X);
81 double R = sqrt( localPosition.
X * localPosition.
X + localPosition.
Y * localPosition.
Y );
106 #if __cplusplus >= 201103L
107 return {rSize, rPhiSize};
109 std::vector<double> cellDims(2,0.0);
111 cellDims[1] = rPhiSize;
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID get(CellID bitfield, size_t idx) const
std::string _rId
the field name used for R
double _offsetR
the coordinate offset in R
virtual ~PolarGridRPhi2()
destructor
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
void set(CellID &bitfield, size_t idx, FieldID value) const
Segmentation base class for polar grids.
PolarGridRPhi2(const std::string &cellEncoding="")
Default constructor passing the encoding string.
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
std::string _description
The description of the segmentation.
double _offsetPhi
the coordinate offset in Phi
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
Namespace for the AIDA detector description toolkit.
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
std::string _type
The segmentation type.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions: d...
std::string _phiId
the field name used for Phi
std::vector< double > _gridPhiValues
the grid sizes in Phi
std::vector< double > _gridRValues
the grid boundaries in R