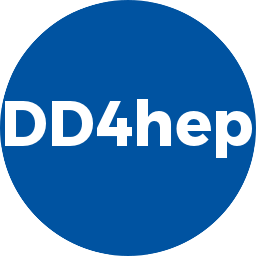 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_MATERIALSCAN_H
14 #define DDREC_MATERIALSCAN_H
88 const MaterialVec&
scan(
double x0,
double y0,
double z0,
double x1,
double y1,
double z1,
double epsilon=1e-4)
const;
94 void print(
double x0,
double y0,
double z0,
95 double x1,
double y1,
double z1,
100 #endif // DDREC_MATERIALSCAN_H
void setDetector(DetElement detector)
Set a specific detector volume to limit the scan (resets other selection criteria)
void setRegion(const char *region)
Set a specific region to limit the scan (resets other selection criteria)
const MaterialVec & scan(double x0, double y0, double z0, double x1, double y1, double z1, double epsilon=1e-4) const
Scan along a line and store the matrials internally.
std::vector< std::pair< Material, double > > MaterialVec
MaterialScan()
Default constructor.
virtual ~MaterialScan()
Default destructor.
Handle class describing a material.
std::set< const TGeoNode * > m_placements
Local cache: subdetector placements.
Handle class describing a detector element.
std::unique_ptr< MaterialManager > m_materialMgr
Material manager.
void setMaterial(const char *material)
Set a specific volume material to limit the scan (resets other selection criteria)
Handle class describing a region as used in simulation.
void print(const Vector3D &start, const Vector3D &end, double epsilon=1e-4) const
Scan along a line and print the materials traversed.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
Detector & m_detector
Reference to detector setup.
Class to perform material scans along a straight line.