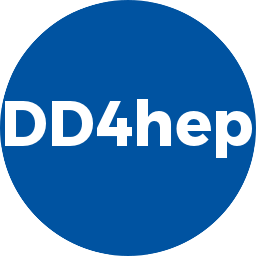 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
30 m_constructSD(), m_constructFLD(), m_constructGEO()
32 m_needsControl =
true;
55 except(
"+++ %s returned %d, not SUCCESS (1). Terminating setup",desc.c_str(), ret);
62 info(
"+++ Worker:%ld Execute PYTHON constructGeo %s....",
70 info(
"+++ Worker:%ld Execute PYTHON constructField %s....",
77 info(
"+++ Worker:%ld Execute PYTHON constructSensitives %s....",
Base class to initialize a multi-threaded or single threaded Geant4 application.
void exec(const std::string &desc, const Geant4PythonCall &cmd) const
Execute command in the python interpreter.
Geant4PythonCall m_constructSD
Sensitive detector initialization command. Default: empty.
virtual void constructField(Geant4DetectorConstructionContext *ctxt) override
Electromagnetic field construction callback. Called at "ConstructSDandField()".
void setConstructSensitives(PyObject *callable, PyObject *args)
Set the sensitive detector initialization command.
Geant4PythonCall m_constructFLD
Field initialization command. Default: empty.
void setConstructGeo(PyObject *callable, PyObject *args)
Set the Detector initialization command.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
void setConstructField(PyObject *callable, PyObject *args)
Set the field initialization command.
bool isValid() const
Check if call is set.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
Base class to initialize a multi-threaded or single threaded Geant4 application.
Geant4PythonCall m_constructGEO
Geometry initialization command. Default: empty.
void set(PyObject *callable, PyObject *args)
Set the callback structures for callbacks with arguments.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
RETURN execute() const
Execute command in the python interpreter.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt) override
Geometry construction callback. Called at "Construct()".
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4PythonDetectorConstruction(Geant4Context *c, const std::string &nam)
Standard constructor.
Basic implementation of the Geant4 detector construction action.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.