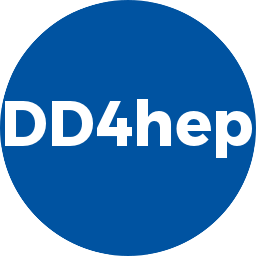 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_PYTHON_DDPYTHON_H
15 #define DDG4_PYTHON_DDPYTHON_H 1
21 #include <TPyReturn.h>
26 struct DDPythonGlobalState;
69 static void assignObject(PyObject*& obj, PyObject* new_obj);
80 int setArgs(
int argc,
char** argv)
const;
81 int runFile(
const std::string& fname)
const;
82 int execute(
const std::string& cmd)
const;
83 int evaluate(
const std::string& cmd)
const;
93 PyObject*
call(PyObject* method, PyObject* args);
104 TPyReturn
callC(PyObject* method, PyObject* args);
117 #endif // DDG4_PYTHON_DDPYTHON_H
DDPython()
Standard constructor.
static bool isMainThread()
TPyReturn callC(PyObject *method, PyObject *args)
Call a python object with argument (typically a dictionary).
static void setMainThread()
Python interface class for callbacks and GIL.
int evaluate(const std::string &cmd) const
static void allowThreads()
Save thread state.
static int run_interpreter(int argc, char **argv)
Start the interpreter in normal mode without hacks like 'python.exe' does.
void afterFork() const
Callback after forking.
static void assignObject(PyObject *&obj, PyObject *new_obj)
Release python object.
static void releaseObject(PyObject *&obj)
Release python object.
int setArgs(int argc, char **argv) const
static void restoreThread()
int execute(const std::string &cmd) const
static DDPython instance()
Object instantiator.
int runFile(const std::string &fname) const
Namespace for the AIDA detector description toolkit.
DDPython(const DDPython &)
Copy constructor.
void prompt() const
Invoke command prompt.
PyObject * call(PyObject *method, PyObject *args)
Call a python object with argument (typically a dictionary)