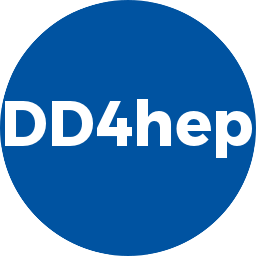 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
32 declareProperty(
"Offset", m_offset);
33 declareProperty(
"Sigma", m_sigma);
34 declareProperty(
"Mask", m_mask = 1);
35 m_needsControl =
true;
52 print(
"+++ Smearing primary vertex for interaction type %d (%d Vertices, %d particles) "
53 "by (%+.2e mm, %+.2e mm, %+.2e mm, %+.2e ns)",
54 m_mask,
int(inter->vertices.size()),
int(inter->particles.size()),dx,dy,dz,dt);
58 print(
"+++ No interaction of type %d present.",
m_mask);
63 typedef std::vector<Geant4PrimaryInteraction*> _I;
72 for(_I::iterator i=interactions.begin(); i != interactions.end(); ++i)
ROOT::Math::PxPyPzEVector m_sigma
Property: The gaussian sigmas to the offset.
Class modelling a complete primary event with multiple interactions.
void smear(Interaction *interaction) const
Action routine to smear one single interaction according to the properties.
std::vector< Geant4PrimaryInteraction * > interactions() const
Retrieve all interactions.
virtual ~Geant4InteractionVertexSmear()
Default destructor.
static void increment(T *)
Increment count according to type information.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
Geant4Random & random() const
Access the random number generator.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
Geant4InteractionVertexSmear()=delete
Inhibit default constructor.
static void decrement(T *)
Decrement count according to type information.
Concrete implementation of the Geant4 generator action base class.
int m_mask
Property: Unique identifier of the interaction created.
Mini interface to THE random generator of the application.
ROOT::Math::PxPyPzEVector m_offset
Property: The constant smearing offset.
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
double gauss(double mean=0, double sigma=1)
Create gaussian distributed random numbers.
int smearInteraction(const Geant4Action *caller, Geant4PrimaryEvent::Interaction *inter, double dx, double dy, double dz, double dt)
Smear the primary vertex of an interaction.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual void operator()(G4Event *event)
Callback to generate primary particles.
Geant4PrimaryInteraction Interaction
Interaction definition.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4PrimaryInteraction * get(int id) const
Retrieve an interaction by its ID.