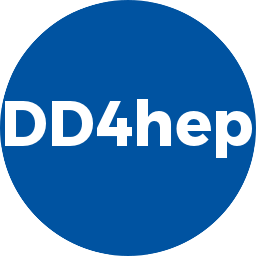 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 #include <G4Threading.hh>
21 #include <G4AutoLock.hh>
26 G4Mutex action_mutex=G4MUTEX_INITIALIZER;
65 throw std::runtime_error(
"Geant4SharedGeneratorAction: Attempt to use invalid actor!");
71 G4AutoLock protection_lock(&action_mutex); {
112 G4AutoLock protection_lock(&action_mutex);
117 except(
"Attempt to add invalid actor!");
122 if (
context()->kernel().processEvents() ) {
123 m_actors(&Geant4GeneratorAction::operator(), event);
CallbackSequence m_calls
Callback sequence to generate primary particles.
bool m_needsControl
Default property: Flag to create control instance.
Actors< Geant4GeneratorAction > m_actors
The list of action objects to be called.
virtual void configureFiber(Geant4Context *thread_context)
Set or update client for the use in a new thread fiber.
Functor to access elements by name.
Geant4Context * m_context
Reference to the Geant4 context.
virtual ~Geant4GeneratorActionSequence()
Default destructor.
Geant4GeneratorAction(Geant4Context *context, const std::string &name)
Standard constructor.
static void increment(T *)
Increment count according to type information.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
Functor to update the context of a Geant4Action object.
Geant4GeneratorActionSequence(Geant4Context *context, const std::string &name)
Standard constructor.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
static void decrement(T *)
Decrement count according to type information.
Concrete implementation of the Geant4 generator action base class.
Geant4GeneratorAction * get(const std::string &name) const
Get an action by name.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
virtual void operator()(G4Event *event)
Callback to generate primary particles.
void clear()
Clear the sequence and remove all callbacks.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
Geant4GeneratorAction * m_action
Reference to the shared action.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual ~Geant4GeneratorAction()
Default destructor.
virtual ~Geant4SharedGeneratorAction()
Default destructor.
virtual void use(Geant4GeneratorAction *action)
Underlying object to be used during the execution of this thread.
Geant4SharedGeneratorAction(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
virtual void operator()(G4Event *event) override
User generator callback.
Helper class to indicate the stop of processing.
Generic context to extend user, run and event information.
void adopt(Geant4GeneratorAction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
Geant4Context * context() const
Access the context.