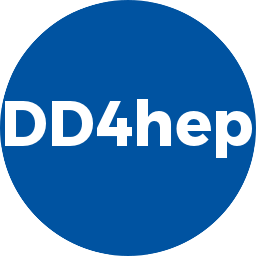 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #include <G4Threading.hh>
20 #include <G4AutoLock.hh>
25 G4Mutex event_action_mutex=G4MUTEX_INITIALIZER;
74 except(
"Geant4SharedEventAction: Attempt to use invalid actor!");
80 G4AutoLock protection_lock(&event_action_mutex); {
90 G4AutoLock protection_lock(&event_action_mutex); {
133 G4AutoLock protection_lock(&event_action_mutex);
138 except(
"Geant4EventActionSequence: Attempt to add invalid actor!");
virtual void begin(const G4Event *event)
Begin-of-event callback.
bool m_needsControl
Default property: Flag to create control instance.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
virtual void configureFiber(Geant4Context *thread_context)
Set or update client for the use in a new thread fiber.
Geant4EventActionSequence(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
Functor to access elements by name.
Geant4Context * m_context
Reference to the Geant4 context.
static void increment(T *)
Increment count according to type information.
Geant4EventAction * m_action
Reference to the shared action.
Functor to update the context of a Geant4Action object.
PropertyManager m_properties
Property pool.
Actors< Geant4EventAction > m_actors
The list of action objects to be called.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Concrete basic implementation of the Geant4 event action.
Geant4EventAction(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void use(Geant4EventAction *action)
Underlying object to be used during the execution of this thread.
void adopt(const PropertyManager ©)
Import properties of another instance.
CallbackSequence m_begin
Callback sequence for event initialization action.
virtual void begin(const G4Event *event)
Begin-of-event callback.
static void decrement(T *)
Decrement count according to type information.
CallbackSequence m_end
Callback sequence for event finalization action.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
void clear()
Clear the sequence and remove all callbacks.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
virtual ~Geant4EventAction()
Default destructor.
PropertyManager & properties()
Access to the properties of the object.
virtual ~Geant4SharedEventAction()
Default destructor.
virtual void end(const G4Event *event)
End-of-event callback.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual void begin(const G4Event *event) override
Begin-of-event callback.
Geant4EventAction * get(const std::string &name) const
Get an action by name.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
CallbackSequence m_final
Callback sequence for event finalization action.
virtual void end(const G4Event *event)
End-of-event callback.
Geant4SharedEventAction(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual ~Geant4EventActionSequence()
Default destructor.
virtual void end(const G4Event *event) override
End-of-event callback.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
void adopt(Geant4EventAction *action)
Add an actor responding to all callbacks. Sequence takes ownership.