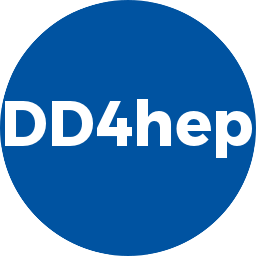 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
27 #include <G4SystemOfUnits.hh>
28 #include <G4FastStep.hh>
52 double mom = momentum/CLHEP::GeV;
78 for( smeared = -1e0; smeared < 0e0; ) {
89 declareProperty(
"StocasticResolution", locals.StocasticEnergyResolution );
90 declareProperty(
"ConstantResolution", locals.ConstantEnergyResolution );
91 declareProperty(
"NoiseResolution", locals.NoiseEnergyResolution );
103 auto* primary = track.GetPrimaryTrack();
105 this->killParticle(step, primary->GetKineticEnergy(), 0e0);
115 if ( !spot.
primary->GetParentID() ) {
116 deposit = locals.smearEnergy(deposit);
119 step.ProposeTotalEnergyDeposited(deposit);
120 this->locals.hitMaker.make(hit, track);
virtual void modelShower(const G4FastTrack &track, G4FastStep &step) override
User callback to model the particle/energy shower.
G4FastSimHitMaker hitMaker
static Geant4Random * instance(bool throw_exception=true)
Access the main Geant4 random generator instance. Must be created before used!
G4ThreeVector trackPosition() const
Primary track position.
double kineticEnergy() const
Primary track kinetic energy.
void SetPosition(const G4ThreeVector &aPosition)
Set position.
double StocasticEnergyResolution
void initialize()
Declare optional properties from embedded structure.
Spot definition for fast simulation and GFlash.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
#define DECLARE_GEANT4ACTION_NS(name_space, name)
Plugin defintion to create Geant4Action objects.
Geant4 detector construction context definition.
Geant4 wrapper for the Geant4 fast simulation shower model.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
double ConstantEnergyResolution
void SetEnergy(const G4double &aEnergy)
Set energy.
Geant4FSShowerModel< calo_smear_model > Geant4CaloSmearShowerModel
double resolution(double momentum) const
double gauss(double mean=0, double sigma=1)
Create gaussian distributed random numbers.
double smearEnergy(double mom) const
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Namespace for the AIDA detector description toolkit.
Configuration structure for the fast simulation shower model Geant4FSShowerModel<par02_em_model>
double NoiseEnergyResolution