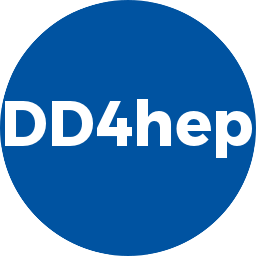 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
35 return Callback(
this).
make(&Geant4PhaseAction::operator());
40 const std::type_info& arg_type1,
const std::type_info& arg_type2)
51 (*i).first->release();
66 Members::iterator i = find(
m_members.begin(),
m_members.end(), std::make_pair(action,callback));
68 (*i).first->release();
76 if ( (*i).first == action && (*i).second.par == callback.
par) {
77 (*i).first->release();
88 (*i).second.execute((
const void**) &argument);
92 class G4HCofThisEvent;
93 class G4TouchableHistory;
108 this->execute((
const void**) &stackManager);
111 template <>
void Geant4ActionPhase::call<const G4Run*>(
const G4Run* run) {
112 this->execute((
const void**) &run);
115 template <>
void Geant4ActionPhase::call<G4Event*>(G4Event* event) {
116 this->execute((
const void**) &event);
119 template <>
void Geant4ActionPhase::call<const G4Event*>(
const G4Event* event) {
120 this->execute((
const void**) &event);
123 template <>
void Geant4ActionPhase::call<const G4Track*>(
const G4Track* track) {
124 this->execute((
const void**) &track);
127 template <>
void Geant4ActionPhase::call<const G4Step*>(
const G4Step* step) {
128 this->execute((
const void**) &step);
131 template <>
void Geant4ActionPhase::call<const G4Step*, G4SteppingManager*>(
const G4Step* step, G4SteppingManager* mgr) {
132 const void * args[] = { step, mgr };
136 template <>
void Geant4ActionPhase::call<G4HCofThisEvent*>(G4HCofThisEvent* hce) {
137 this->execute((
const void**) &hce);
140 template <>
void Geant4ActionPhase::call<G4Step*, G4TouchableHistory*>(G4Step* step, G4TouchableHistory* history) {
141 const void * args[] = { step, history };
static void increment(T *)
Increment count according to type information.
Geant4PhaseAction(Geant4Context *context, const std::string &name)
Standard constructor.
Members m_members
Phase members (actions) being called for a particular phase.
const Callback & make(R(T::*pmf)())
Callback setup function for Callbacks with member functions with explicit return type taking no argum...
virtual bool add(Geant4Action *action, Callback callback)
Add a new member to the phase.
virtual void operator()()
Callback to generate primary particles.
Definition of the generic callback structure for member functions.
static void decrement(T *)
Decrement count according to type information.
virtual Callback callback()
Create bound callback to operator()()
Default base class for all Geant 4 actions and derivates thereof.
void call()
Create action to execute phase members.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
void execute(void *argument)
Execute all members in the phase context.
Namespace for the AIDA detector description toolkit.
virtual bool remove(Geant4Action *action, Callback callback)
Remove an existing member from the phase. If not existing returns false.
Geant4ActionPhase(Geant4Context *context, const std::string &name, const std::type_info &arg_type0, const std::type_info &arg_type1, const std::type_info &arg_type2)
Standard constructor.
const std::type_info * m_argTypes[3]
Type information of the argument type of the callback.
virtual ~Geant4PhaseAction()
Default destructor.
virtual ~Geant4ActionPhase()
Default destructor.
Generic context to extend user, run and event information.