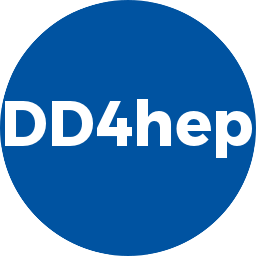 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #include <xercesc/dom/DOMException.hpp>
59 throw std::runtime_error(
"dd4hep: Failed to parse the XML file " + xmlfile +
" [Invalid XML ROOT handle]");
62 throw std::runtime_error(
xml::_toString(e.msg) +
"\ndd4hep: XML-DOM Exception while parsing " + xmlfile);
65 throw std::runtime_error(std::string(e.what()) +
"\ndd4hep: while parsing " + xmlfile);
68 throw std::runtime_error(
"dd4hep: UNKNOWN exception while parsing " + xmlfile);
84 throw std::runtime_error(
"dd4hep: Failed to parse the XML file " + xmlfile +
" [Invalid XML ROOT handle]");
87 throw std::runtime_error(
xml::_toString(e.msg) +
"\ndd4hep: XML-DOM Exception while parsing " + xmlfile);
90 throw std::runtime_error(std::string(e.what()) +
"\ndd4hep: while parsing " + xmlfile);
93 throw std::runtime_error(
"dd4hep: UNKNOWN exception while parsing " + xmlfile);
115 throw std::runtime_error(
"DetectorLoad::processXMLString: Invalid XML In-memory source [NULL]");
118 throw std::runtime_error(
xml::_toString(e.msg) +
"\ndd4hep: XML-DOM Exception while parsing XML in-memory string.");
121 throw std::runtime_error(std::string(e.what()) +
"\ndd4hep: while parsing XML in-memory string.");
124 throw std::runtime_error(
"dd4hep: UNKNOWN exception while parsing XML in-memory string.");
130 if ( xml_root.
ptr() ) {
131 std::string tag = xml_root.
tag();
132 std::string type = tag +
"_XML_reader";
139 throw std::runtime_error(
"dd4hep: Failed to locate plugin to interprete files of type"
140 " \"" + tag +
"\" - no factory:" + type +
". " + dbg.
missingFactory(type));
143 result = *(
long*) result;
145 throw std::runtime_error(
"dd4hep: Failed to parse the XML file " + xmlfile +
" with the plugin " + type);
149 throw std::runtime_error(
"dd4hep: Failed to parse the XML file " + xmlfile +
" [Invalid XML ROOT handle]");
154 if ( xml_root.
ptr() ) {
155 std::string tag = xml_root.
tag();
156 std::string type = tag +
"_XML_reader";
163 throw std::runtime_error(
"dd4hep: Failed to locate plugin to interprete files of type"
164 " \"" + tag +
"\" - no factory:"
168 result = *(
long*) result;
170 throw std::runtime_error(
"dd4hep: Failed to parse the XML element with tag "
171 + tag +
" with the plugin " + type);
175 throw std::runtime_error(
"dd4hep: Failed to parse the XML file [Invalid XML ROOT handle]");
std::string tag() const
Text access to the element's tag.
xercesc::DOMException XmlException
void exception(const std::string &src, const std::string &msg)
Detector * m_detDesc
Reference to the Detector instance.
virtual ~DetectorLoad()
Default destructor.
Class to easily access the properties of single XmlElements.
virtual void processXMLElement(const std::string &msg_source, const xml::Handle_t &root)
Process a given DOM (sub-) tree.
void parse(Handle_t e, RotationZYX &rot)
Convert rotation XML objects to dd4hep::RotationZYX.
virtual void processXMLString(const char *xmldata)
Process XML unit and adopt all data from source string in momory.
Namespace for the AIDA detector description toolkit supporting XML utilities.
Helper to debug plugin manager calls.
std::string _toString(const Attribute attr)
Convert xml attribute to STL string.
DetectorLoad()=delete
No defautl constructor.
Helper class to encapsulate a unicode string.
std::string missingFactory(const std::string &name) const
Helper to check factory existence.
Class supporting the basic functionality of an XML document including ownership.
Class supporting to read data given a URI.
Class supporting to read and parse XML documents.
DetectorBuildType
Detector description build types.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
virtual void processXML(const std::string &fname, xml::UriReader *entity_resolver=0)
Process XML unit and adopt all data from source structure.
Elt_t ptr() const
Direct access to the XmlElement by function.
void handle(const O *o, const C &c, F pmf)
Handle_t root() const
Access the ROOT eleemnt of the DOM document.