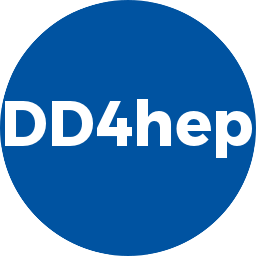 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
43 struct DetectorHelperTest {
45 DetectorHelperTest(
Detector& description,
int argc,
char** argv) {
47 const char* nam = argc>1 ? argv[1]+1 :
"SiVertexEndcap";
52 virtual ~DetectorHelperTest() {}
56 for(DetElement::Children::const_iterator i=de.
children().begin(); i!=de.
children().end(); ++i) {
59 if ( child.
children().size() > 0 ) walkSD(h,child);
64 printout(INFO,
"DetectorHelperTest",
"Sensitive detector[%s]: %p --> %s",de.
path().c_str(),(
void*)sd.
ptr(),
70 printout(INFO,
"DetectorHelperTest",
"Sensitive detector[%s]: %p --> %s",nam,(
void*)sd.
ptr(),
75 static long run(
Detector& description,
int argc,
char** argv) {
76 DetectorHelperTest test(description,argc,argv);
83 using ::DetectorHelperTest;
85 DECLARE_APPLY(CLICSiD_DetectorHelperTest,DetectorHelperTest::run)
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
virtual DetElement detector(const std::string &name) const =0
Retrieve a subdetector element by its name from the detector description.
Handle class to hold the information of a sensitive detector.
#define DECLARE_APPLY(name, func)
const char * name() const
Access the object name (or "" if not supported by the object)
Handle class describing a detector element.
DetectorHelper: class to shortcut certain questions to the dd4hep detector description interface.
SensitiveDetector sensitiveDetector(const std::string &detector) const
Access the sensitive detector of a given subdetector (if the sub-detector is sensitive!...
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.