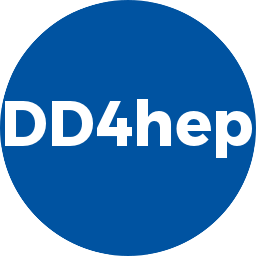 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
39 std::string path = x_det.
attr<std::string>(
_U(path));
41 if (
det.isValid() ) {
42 std::size_t count = 0;
43 bool propagate =
det == detector.
world() ?
false: x_det.attr<
bool>(
_U(propagate),
false);
44 if (
xml_dim_t x_vol = x_det.child(
_U(volume),
false) ) {
48 printout(lvl,
"DetElementConfig",
"++ Applied %ld settings to %s", count, path.c_str());
51 except(
"DetElementConfig",
"FAILED: No valid DetElement. Configuration could not be applied!");
64 std::string name = c.
attr<std::string>(
_U(detector));
67 printout(lvl,
"SensDetConfig",
"++ Applied %ld settings to %s", count, name.c_str());
std::size_t configVolume(dd4hep::Detector &detector, dd4hep::xml::Handle_t element, dd4hep::Volume volume, bool propagate, bool ignore_unknown_attr=false)
Configure volume properties from XML element.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
const XmlAttr * Attribute
std::size_t debug(const std::string &src, const std::string &msg)
Class to easily access the properties of single XmlElements.
dd4hep::xml::Component xml_comp_t
Handle class describing a detector element.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
#define DECLARE_XML_PLUGIN(name, func)
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
dd4hep::xml::Dimension xml_dim_t
std::size_t configSensitiveDetector(dd4hep::Detector &detector, dd4hep::SensitiveDetector sensitive, dd4hep::xml::Handle_t element)
Configure sensitive detector from XML element.