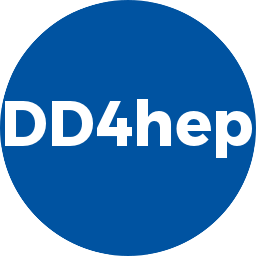 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
38 : lower(low), upper(up), processor(p) {}
40 virtual int process(
Condition c)
const override {
42 if ( h >= lower && h <= upper )
51 Scanner scn(low,up,processor);
55 dd4hep::except(
"ConditionsMap",
"Cannot scan conditions map for conditions of an invalid detector element!");
70 std::vector<Condition>& result;
74 virtual int process(
Condition c)
const override {
76 if ( h >= lower && h <= upper ) {
77 result.emplace_back(c);
83 std::vector<Condition> result;
88 Scanner scn(low,up,result);
92 dd4hep::except(
"ConditionsMap",
"Cannot scan conditions map for conditions of an invalid detector element!");
104 template <
typename T>
107 return (res == data.end()) ?
Condition() : res->second;
111 template <
typename T>
113 for(
const auto& i : data )
118 template <
typename T>
127 typename T::const_iterator first = data.lower_bound(low);
128 for(; first != data.end() && (*first).first <= up; ++first )
129 processor((*first).second);
132 dd4hep::except(
"ConditionsMap",
"Cannot scan conditions map for conditions of an invalid detector element!");
Concrete ConditionsMap implementation class using externally defined containers.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
virtual void scan(const Condition::Processor &processor) const =0
Interface to scan data content of the conditions mapping.
bool isValid() const
Check the validity of the object held by the handle.
Key definition to optimize ans simplyfy the access to conditions entities.
Helper union to interprete conditions keys.
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition) override
Insert a new entry to the map.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
Handle class describing a detector element.
virtual void scan(const Condition::Processor &processor) const override
Interface to scan data content of the conditions mapping.
virtual Condition get(DetElement detector, Condition::itemkey_type key) const =0
Interface to access conditions by hash value. The detector element key and the item key make a unique...
unsigned int itemkey_type
Low part of the key identifies the item identifier.
unsigned long long int key_type
Forward definition of the key type.
Namespace for the AIDA detector description toolkit.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
Abstract base for processing callbacks to conditions objects.
virtual Condition get(DetElement detector, Condition::itemkey_type key) const override
Interface to access conditions by hash value.