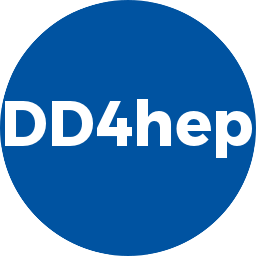 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 namespace DDSegmentation {
27 _type =
"WaferGridXY";
28 _description =
"Cartesian segmentation in the local XY-plane for both Normal wafer and Magic wafer(depending on the layer dimensions)";
46 _type =
"WaferGridXY";
47 _description =
"Cartesian segmentation in the local XY-plane for both Normal wafer and Magic wafer(depending on the layer dimensions)";
69 unsigned int _groupMGWaferIndex;
70 unsigned int _waferIndex;
99 unsigned int _groupMGWaferIndex;
100 unsigned int _waferIndex;
129 #if __cplusplus >= 201103L
132 std::vector<double> cellDims(2,0.0);
std::string _identifierWafer
encoding field used for the wafer
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
Simple container for a physics vector.
double _waferOffsetY[MAX_GROUPS][MAX_WAFERS]
list of wafer y offset for each group
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
std::string _yId
the field name used for Y
FieldID get(CellID bitfield, size_t idx) const
double _offsetX
the coordinate offset in X
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
double _offsetY
the coordinate offset in Y
std::string _identifierMGWaferGroup
encoding field used for the Magic Wafer group
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
double _waferOffsetX[MAX_GROUPS][MAX_WAFERS]
list of wafer x offset for each group
virtual ~WaferGridXY()
destructor
void set(CellID &bitfield, size_t idx, FieldID value) const
WaferGridXY(const std::string &cellEncoding="")
Default constructor passing the encoding string.
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
double _gridSizeX
the grid size in X
std::string _description
The description of the segmentation.
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
Namespace for the AIDA detector description toolkit.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
std::string _xId
the field name used for X
std::string _type
The segmentation type.
double _gridSizeY
the grid size in Y
Segmentation base class describing cartesian grid segmentation.