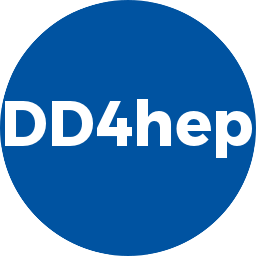 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <TEveManager.h>
22 #include <TEveBrowser.h>
23 #include <TEveWindow.h>
24 #include <TGLViewer.h>
30 :
View(eve, nam), m_projMgr(0), m_axis(0)
52 if ( el == unprojected ) {
57 TEveElement* e =
m_projMgr->ImportElements(el, list);
58 printout(INFO,
"Projection",
"ImportElement %s [%s] into list: %s Projectable:%s [%p]",
60 dynamic_cast<TEveProjectable*
>(list) ?
"true" :
"false", e);
62 unprojected->AddElement(el);
84 TEveProjectionAxes* a =
new TEveProjectionAxes(
m_projMgr);
85 a->SetMainColor(kWhite);
87 a->SetTitleSize(0.05);
89 a->SetLabelSize(0.025);
99 m_projMgr =
new TEveProjectionManager(TEveProjection::kPT_RPhi);
108 m_projMgr =
new TEveProjectionManager(TEveProjection::kPT_RhoZ);
117 m_view->GetGLViewer()->SetCurrentCamera(TGLViewer::kCameraOrthoXOY);
The main class of the DDEve display.
virtual TEveElement * ImportGeoTopic(TEveElement *element, TEveElementList *list) override
Call an element to a geometry element list.
TEveProjectionAxes * m_axis
Reference to the projection axis.
virtual View & Map(TEveWindow *slot)
Map the view view to the slot.
virtual TEveElement * ImportEventElement(TEveElement *element, TEveElementList *list) override
Call an element to a event element list.
virtual Projection & AddAxis()
Add projection axis to the view.
class Projection Projection.h DDEve/Projection.h
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
virtual TEveElementList & GetGeoTopic(const std::string &name)
Access/Create an geometry topic by name.
const std::string & name() const
Access to the view name/title.
const char * GetName(T *p)
virtual TEveElementList * AddToGlobalItems(const std::string &nam)
Add the view to the global list of eve objects.
View TEveWindowSlot * slot
virtual TEveElement * ImportGeoElement(TEveElement *element, TEveElementList *list) override
Call an element to a geometry element list.
TEveScene * m_geoScene
Reference to the geometry scene.
virtual ~Projection()
Default destructor.
virtual void SetDepth(Float_t d)
virtual TEveElement * ImportElement(TEveElement *el, TEveElementList *list)
Call an element to a event element list.
virtual View & Map(TEveWindow *slot) override
Map the projection view to the slot.
TEveViewer * m_view
Reference to the view.
Namespace for the AIDA detector description toolkit.
virtual Projection & CreateRhoPhiProjection()
Create Rho-Phi projection.
class View View.h DDEve/View.h
TEveProjectionManager * m_projMgr
Reference to the projection manager.
TEveScene * m_eveScene
Reference to the event scene.
Projection(Display *eve, const std::string &name)
Initializing constructor.
virtual Projection & CreateRhoZProjection()
Create Rho-Z projection.