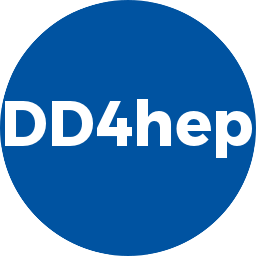 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDEVE_PROJECTION_H
14 #define DDEVE_PROJECTION_H
20 #include <TEveProjectionManager.h>
21 #include <TEveProjectionAxes.h>
68 #endif // DDEVE_PROJECTION_H
The main class of the DDEve display.
virtual TEveElement * ImportGeoTopic(TEveElement *element, TEveElementList *list) override
Call an element to a geometry element list.
TEveProjectionAxes * m_axis
Reference to the projection axis.
virtual TEveElement * ImportEventElement(TEveElement *element, TEveElementList *list) override
Call an element to a event element list.
virtual Projection & AddAxis()
Add projection axis to the view.
class Projection Projection.h DDEve/Projection.h
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
const std::string & name() const
Access to the view name/title.
View TEveWindowSlot * slot
virtual TEveElement * ImportGeoElement(TEveElement *element, TEveElementList *list) override
Call an element to a geometry element list.
virtual ~Projection()
Default destructor.
virtual void SetDepth(Float_t d)
virtual TEveElement * ImportElement(TEveElement *el, TEveElementList *list)
Call an element to a event element list.
virtual View & Map(TEveWindow *slot) override
Map the projection view to the slot.
Namespace for the AIDA detector description toolkit.
virtual Projection & CreateRhoPhiProjection()
Create Rho-Phi projection.
class View View.h DDEve/View.h
TEveProjectionManager * m_projMgr
Reference to the projection manager.
ClassDefOverride(Projection, 0)
Projection(Display *eve, const std::string &name)
Initializing constructor.
virtual Projection & CreateRhoZProjection()
Create Rho-Z projection.