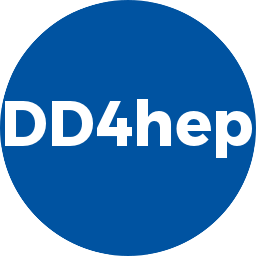 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 #include <IO/LCReader.h>
21 #include <EVENT/LCCollection.h>
22 #include <EVENT/SimCalorimeterHit.h>
23 #include <EVENT/SimTrackerHit.h>
24 #include <EVENT/MCParticle.h>
35 using namespace EVENT;
39 const double* p = hit->getPosition();
43 target->
deposit = hit->getEDep();
47 const float* p = hit->getPosition();
51 target->
deposit = hit->getEnergy();
55 static const void* _convertHitFunc(
const LCObject* source,
DDEveHit* target) {
56 const SimTrackerHit* t =
dynamic_cast<const SimTrackerHit*
>(source);
57 if (t &&
_fill(t,target))
return t;
58 const SimCalorimeterHit* c =
dynamic_cast<const SimCalorimeterHit*
>(source);
59 if (c &&
_fill(c,target))
return c;
62 static const void* _convertParticleFunc(
const LCObject* source,
DDEveParticle* target) {
63 if ( source && target ) {}
67 static void* _create(
const char*) {
74 LCIOEventHandler::LCIOEventHandler() :
EventHandler(), m_lcReader(0), m_event(0) {
76 m_lcReader = LCFactory::getInstance()->createLCReader() ;
80 LCIOEventHandler::~LCIOEventHandler() {
105 Branches::const_iterator ibr =
m_branches.find(collection);
107 LCCollection* c = (*ibr).second;
110 int n = c->getNumberOfElements();
112 for(
int i=0; i<n; ++i) {
113 LCObject* ptr = c->getElementAt(i);
114 if ( _convertHitFunc(ptr,&hit) ) {
126 Branches::const_iterator ibr =
m_branches.find(collection);
128 LCCollection* c = (*ibr).second;
131 int n = c->getNumberOfElements();
133 for(
int i=0; i<n; ++i) {
134 LCObject* ptr = c->getElementAt(i);
135 if ( _convertParticleFunc(ptr,&part) ) {
166 typedef std::vector<std::string> _S;
167 const _S* collnames =
m_event->getCollectionNames();
168 for( _S::const_iterator i = collnames->begin(); i != collnames->end(); ++i) {
169 LCCollection* c =
m_event->getCollection(*i);
170 m_data[c->getTypeName()].push_back(std::make_pair((*i).c_str(),c->getNumberOfElements()));
176 throw std::runtime_error(
"+++ EventHandler::readEvent: Failed to read event");
178 throw std::runtime_error(
"+++ EventHandler::readEvent: No file open!");
183 throw std::runtime_error(
"+++ This version of the LCIO reader can only access files sequentially!\n"
184 "+++ Access to the previous event is not supported.");
189 throw std::runtime_error(
"+++ This version of the LCIO reader can only access files sequentially!\n"
190 "+++ Random access is not supported.");
Event data actor base class for particles. Used to extract data from concrete classes.
DDEve event classes: Basic hit.
Branches m_branches
Branch map.
bool m_hasEvent
Flag to indicate that an event is loaded.
Event handler base class: Interface to all DDEve I/O actions.
virtual bool Open(const std::string &type, const std::string &file_name)
Open new data file.
virtual long numEvents() const
Access the number of events on the current input data source (-1 if no data source connected)
Event data actor base class for hits. Used to extract data from concrete classes.
LCIO namespace. See http://lcio.desy.de.
bool m_hasFile
Flag to indicate that a file is opened.
lcio::LCEvent * m_event
Reference to the current LCIO event.
virtual bool GotoEvent(long event_number)
Goto a specified event in the file.
#define DECLARE_CONSTRUCTOR(name, func)
virtual bool hasFile() const
Check if a data file is connected to the handler.
DD4hep internal namespace.
float deposit
Energy deposit.
virtual bool NextEvent()
User overloadable function: Load the next event.
virtual void setSize(size_t)
TypedEventCollections m_data
Data collection map.
const void * _fill(const SimTrackerHit *hit, DDEveHit *target)
Namespace for the AIDA detector description toolkit.
LCIO input event handler for DDEve: Interface class for event I/O.
virtual bool PreviousEvent()
User overloadable function: Load the previous event.
LCIO namespace. See http://lcio.desy.de.
lcio::LCReader * m_lcReader
Reference to data file reader.
Data structure to store the MC particle information.
virtual CollectionType collectionType(const std::string &collection) const
Access to the collection type by name.
virtual void setSize(size_t)
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor)
Call functor on hit collection.