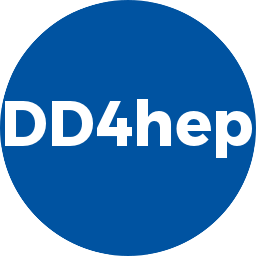 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDEVE_LCIO_LCIOEVENTHANDLER_H
14 #define DDEVE_LCIO_LCIOEVENTHANDLER_H
39 typedef std::map<std::string,lcio::LCCollection*>
Branches;
40 typedef const void* (*HitAccessor_t)(
const lcio::LCObject*,
DDEveHit*);
72 virtual bool Open(
const std::string& type,
const std::string& file_name);
78 virtual bool GotoEvent(
long event_number);
83 #endif // DDEVE_LCIO_LCIOEVENTHANDLER_H
Event data actor base class for particles. Used to extract data from concrete classes.
DDEve event classes: Basic hit.
std::map< std::string, lcio::LCCollection * > Branches
Branches m_branches
Branch map.
std::string datasourceName() const
Access the data source name.
Event handler base class: Interface to all DDEve I/O actions.
virtual bool Open(const std::string &type, const std::string &file_name)
Open new data file.
virtual long numEvents() const
Access the number of events on the current input data source (-1 if no data source connected)
Event data actor base class for hits. Used to extract data from concrete classes.
lcio::LCEvent * m_event
Reference to the current LCIO event.
virtual bool GotoEvent(long event_number)
Goto a specified event in the file.
virtual bool hasFile() const
Check if a data file is connected to the handler.
virtual bool NextEvent()
User overloadable function: Load the next event.
LCIOEventHandler()
Standard constructor.
virtual const TypedEventCollections & data() const
Access the map of simulation data collections.
TypedEventCollections m_data
Data collection map.
Namespace for the AIDA detector description toolkit.
LCIO input event handler for DDEve: Interface class for event I/O.
virtual bool PreviousEvent()
User overloadable function: Load the previous event.
virtual ~LCIOEventHandler()
Default destructor.
lcio::LCReader * m_lcReader
Reference to data file reader.
std::map< std::string, std::vector< Collection > > TypedEventCollections
Types collection: collections are grouped by type (class name)
std::string m_fileName
Input file name.
virtual CollectionType collectionType(const std::string &collection) const
Access to the collection type by name.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor)
Call functor on hit collection.