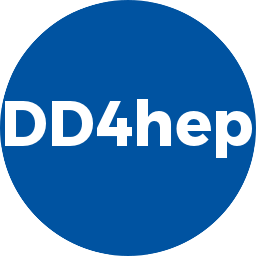 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 namespace DDSegmentation {
25 _description =
"Hexagonal segmentation in the local XY-plane";
41 _description =
"Hexagonal segmentation in the local XY-plane";
90 return std::fmod(std::fmod(i,n) + n,n);
126 (b<0.5)*(-std::abs(a-.5)<(b-.5)*3)+(b>0.5)*(std::abs(a-.5)-.5<(b-1)*3);
127 int iy=std::floor(y/(std::sqrt(3)*
_sideLength/2.));
136 #if __cplusplus >= 201103L
139 std::vector<double> cellDims(2,0.0);
std::string _staggerKeyword
the keyword used to determine which volumes to stagger
std::string _yId
the field name used for Y
double positive_modulo(double i, double n)
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID get(CellID bitfield, size_t idx) const
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
HexGrid(const std::string &cellEncoding="")
Default constructor used by derived classes passing the encoding string.
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
int _stagger
the stagger mode: 0=off ; 1=cycle through 3 different offsets (H3)
std::string _xId
the field name used for X
void set(CellID &bitfield, size_t idx, FieldID value) const
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
std::string _description
The description of the segmentation.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
double _sideLength
the length of one side of a hexagon
virtual ~HexGrid()
Destructor.
Namespace for the AIDA detector description toolkit.
std::string _type
The segmentation type.
double _offsetY
the coordinate offset in Y
Base class for all segmentations.
double _offsetX
the coordinate offset in X