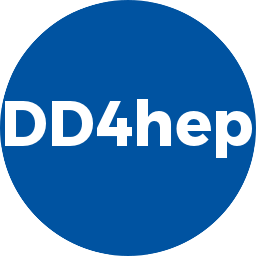 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_HEXGRID_H
20 #define DDSEGMENTATION_HEXGRID_H
25 namespace DDSegmentation {
34 HexGrid(
const std::string& cellEncoding =
"");
131 #endif // DDSEGMENTATION_HEXGRID_H
std::string _staggerKeyword
the keyword used to determine which volumes to stagger
std::string _yId
the field name used for Y
void setFieldNameY(const std::string &fieldName)
set the field name used for Y
const std::string & fieldNameX() const
access the field name used for X
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
const std::string & staggerKeyword() const
access the keyword for staggering
void setStaggerKeyword(const std::string &staggerKeyword)
set the keyword used to determine which volumes to stagger
HexGrid(const std::string &cellEncoding="")
Default constructor used by derived classes passing the encoding string.
void setFieldNameX(const std::string &fieldName)
set the field name used for X
void setStagger(int stagger)
set the stagger mode: 0=no stagger; 1=stagger cycling through 3 offsets
int _stagger
the stagger mode: 0=off ; 1=cycle through 3 different offsets (H3)
void setSideLength(double cellSize)
set the grid size in X
std::string _xId
the field name used for X
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
const std::string & fieldNameY() const
access the field name used for Y
void setOffsetY(double offset)
set the coordinate offset in Y
double offsetX() const
access the coordinate offset in X
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
double _sideLength
the length of one side of a hexagon
double sideLength() const
access the grid size
virtual ~HexGrid()
Destructor.
Namespace for the AIDA detector description toolkit.
double _offsetY
the coordinate offset in Y
double offsetY() const
access the coordinate offset in Y
Base class for all segmentations.
Segmentation base class describing hexagonal grid segmentation, with or without staggering.
double _offsetX
the coordinate offset in X
void setOffsetX(double offset)
set the coordinate offset in X