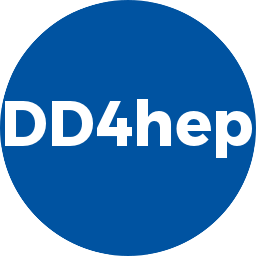 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETAIL_SHAPESINTERNA_H
14 #define DD4HEP_DETAIL_SHAPESINTERNA_H
76 #endif // DD4HEP_DETAIL_SHAPESINTERNA_H
int GetNsegments() const
Access the number of segments.
double GetPhiTwist() const
Access twist angle.
double GetNegativeEndZ() const
Access the negative z.
TwistedTubeObject(const char *pName, double twistedangle, double endinnerrad, double endouterrad, double negativeEndz, double positiveEndz, int nseg, double totphi)
Initializing constructor.
TwistedTubeObject(TwistedTubeObject &&)=delete
Inhibit move constructor.
double GetPositiveEndZ() const
Access the positive z.
TwistedTubeObject()=default
Standard constructor.
Concrete object implementation for the Header handle.
TwistedTubeObject(const TwistedTubeObject &)=delete
Inhibit copy constructor.
virtual void InspectShape() const override
print shape parameters
TwistedTubeObject & operator=(TwistedTubeObject &&)=delete
Inhibit move assignment.
TwistedTubeObject & operator=(const TwistedTubeObject &)=delete
Inhibit copy assignment.
ClassDefOverride(TwistedTubeObject, 0)
virtual ~TwistedTubeObject()=default
Default destructor.
Namespace for the AIDA detector description toolkit.
virtual TGeoShape * GetMakeRuntimeShape(TGeoShape *mother, TGeoMatrix *mat) const override
in case shape has some negative parameters, these has to be computed in order to fit the mother