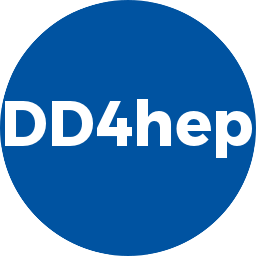 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 class G4ParticleDefinition;
47 const G4ParticleDefinition*
definition()
const;
53 const G4Track*
getTrack(
const G4Step* step)
const {
54 return step->GetTrack();
61 const G4Track*
getTrack(
const G4FastTrack* fast)
const {
62 return fast->GetPrimaryTrack();
78 virtual bool operator()(
const G4Step* step)
const override final {
99 virtual bool operator()(
const G4Step* step)
const override final {
120 virtual bool operator()(
const G4Step* step)
const override final {
144 virtual bool operator()(
const G4Step* step)
const override final {
145 return step->GetTotalEnergyDeposit() >
m_energyCut;
160 #include <G4ParticleTable.hh>
161 #include <G4ChargedGeantino.hh>
162 #include <G4Geantino.hh>
163 #include <G4Track.hh>
192 throw std::runtime_error(
"Invalid particle name:'"+
m_particle+
"' [Not-in-particle-table]");
199 G4ParticleDefinition* def = track->GetDefinition();
206 G4ParticleDefinition* def = track->GetDefinition();
207 if ( def == G4ChargedGeantino::Definition() )
209 if ( def == G4Geantino::Definition() ) {
GeantinoRejectFilter(Geant4Context *c, const std::string &n)
Constructor.
const G4Track * getTrack(const Geant4FastSimSpot *spot) const
Access to the track from step.
virtual ~EnergyDepositMinimumCut()
Standard destructor.
virtual bool operator()(const G4Step *step) const override final
Filter action. Return true if hits should be processed.
Geant4 sensitive detector filter implementing a particle rejector.
const G4ParticleDefinition * definition() const
Safe access to the definition.
virtual bool operator()(const G4Step *step) const override final
Filter action. Return true if hits should be processed.
virtual bool operator()(const Geant4FastSimSpot *spot) const override final
GFlash/FastSim interface: Filter action. Return true if hits should be processed.
Geant4 sensitive detector filter implementing a Geantino rejector.
static void increment(T *)
Increment count according to type information.
EnergyDepositMinimumCut(Geant4Context *c, const std::string &n)
Constructor.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
ParticleFilter(Geant4Context *context, const std::string &name)
Constructor.
Geant4 sensitive detector filter implementing an energy cut.
std::string m_particle
name of the particle to be filtered
Spot definition for fast simulation and GFlash.
double m_energyCut
Energy cut value.
virtual ~ParticleFilter()
Standard destructor.
virtual ~ParticleRejectFilter()
Standard destructor.
const G4Track * getTrack(const G4Step *step) const
Access to the track from step.
bool isGeantino(const G4Track *track) const
Check if the particle is a geantino.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
ParticleRejectFilter(Geant4Context *c, const std::string &n)
Constructor.
static void decrement(T *)
Decrement count according to type information.
virtual bool operator()(const Geant4FastSimSpot *spot) const override final
GFlash/FastSim interface: Filter action. Return true if hits should be processed.
const std::string & name() const
Access name of the action.
virtual bool operator()(const G4Step *step) const override final
Filter action. Return true if hits should be processed.
Geant4 sensitive detector filter implementing a particle selector.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual bool operator()(const Geant4FastSimSpot *spot) const override final
GFlash/FastSim interface: Filter action. Return true if hits should be processed.
virtual ~ParticleSelectFilter()
Standard destructor.
virtual bool operator()(const G4Step *step) const override final
Filter action. Return true if hits should be processed.
Namespace for the AIDA detector description toolkit.
const G4Track * getTrack(const G4FastTrack *fast) const
Access originator track from G4 fast track.
Base class to construct filters for Geant4 sensitive detectors.
bool isSameType(const G4Track *track) const
Check if a track is of the same type.
virtual ~GeantinoRejectFilter()
Standard destructor.
virtual bool operator()(const Geant4FastSimSpot *spot) const override final
GFlash/FastSim interface: Filter action. Return true if hits should be processed.
Geant4 sensitive detector filter base class for particle filters.
G4ParticleDefinition * m_definition
Corresponding geant4 particle definiton.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
ParticleSelectFilter(Geant4Context *c, const std::string &n)
Constructor.