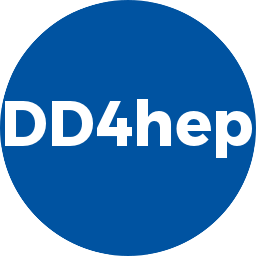 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
25 namespace DDSegmentation {
29 :
Segmentation(cellEncoding), m_discriminator(0), m_debug(0)
32 _type =
"MultiSegmentation";
35 registerParameter<std::string>(
"key",
"Diskriminating field",
m_discriminatorId,
"");
43 _type =
"MultiSegmentation";
46 registerParameter<std::string>(
"key",
"Diskriminating field",
m_discriminatorId,
"");
52 delete (*i).segmentation;
69 (*i).segmentation->setDecoder(newDecoder);
82 printout(ALWAYS,
"MultiSegmentation",
"Id: %04X %s", seg_id, s->
name().c_str());
84 for(
const auto* p : pars ) {
85 printout(ALWAYS,
"MultiSegmentation",
" Param %s = %s",
86 p->name().c_str(), p->value().c_str());
93 except(
"MultiSegmentation",
"Invalid sub-segmentation identifier!");
94 throw std::string(
"Invalid sub-segmentation identifier!");
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID value(CellID bitfield) const
calculate this field's value given an external 64 bit bitmap
Helper structure to describe a sub-segmentation entry.
virtual void addSubsegmentation(long key_min, long key_max, Segmentation *entry)
Add subsegmentation.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
virtual void setDecoder(const BitFieldCoder *decoder)
Set the underlying decoder.
virtual const std::string & name() const
Access the segmentation name.
const Segmentation & subsegmentation(const CellID &cellID) const
Access subsegmentation by cell identifier.
Segmentation * segmentation
virtual Parameters parameters() const
Access to all parameters.
std::vector< Parameter > Parameters
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
Segmentations m_segmentations
Sub-segmentaion container.
virtual ~MultiSegmentation()
Default destructor.
virtual void setDecoder(const BitFieldCoder *decoder)
Set the underlying decoder.
std::string _description
The description of the segmentation.
std::string m_discriminatorId
the field name used to discriminate sub-segmentations
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const =0
Determine the cell ID based on the position.
Namespace for the AIDA detector description toolkit.
MultiSegmentation(const std::string &cellEncoding="")
Default constructor passing the encoding string.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
std::string _type
The segmentation type.
const BitFieldElement * m_discriminator
Bitfield corresponding to dicriminator identifier.
Base class for all segmentations.
virtual Vector3D position(const CellID &cellID) const =0
Determine the local position based on the cell ID.