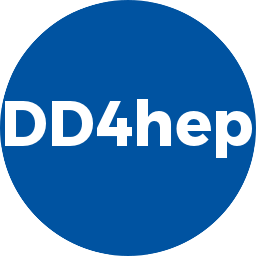 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 namespace DDSegmentation {
25 _type =
"CartesianGridXYZ";
26 _description =
"Cartesian segmentation in the local coordinates";
38 _type =
"CartesianGridXYZ";
39 _description =
"Cartesian segmentation in the local coordinates";
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
double _offsetY
the coordinate offset in Y
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
Segmentation base class describing cartesian grid segmentation in the X-Y plane.
std::string _yId
the field name used for Y
Simple container for a physics vector.
double _gridSizeY
the grid size in Y
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID get(CellID bitfield, size_t idx) const
CartesianGridXYZ(const std::string &cellEncoding)
default constructor using an arbitrary type
double _offsetX
the coordinate offset in X
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
double _offsetZ
the coordinate offset in Z
void set(CellID &bitfield, size_t idx, FieldID value) const
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
std::string _zId
the field name used for Z
virtual ~CartesianGridXYZ()
destructor
std::string _description
The description of the segmentation.
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
Namespace for the AIDA detector description toolkit.
std::string _type
The segmentation type.
double _gridSizeX
the grid size in X
std::string _xId
the field name used for X
double _gridSizeZ
the grid size in Z
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...