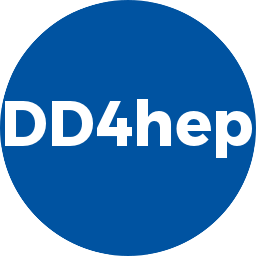 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
31 return (e.
hasAttr(attr_name)) ? e.
attr<T>(attr_name) : std::move(default_value);
43 template <
typename T>
inline std::string
_toString(T value,
const char* fmt) {
49 #endif // XML_HELPER_H
std::string _toString(bool value)
String conversions: boolean value to string.
T getAttrOrDefault(const dd4hep::xml::Element &e, const dd4hep::xml::XmlChar *attr_name, T default_value)
std::string _toString(const Attribute attr)
Convert xml attribute to STL string.
bool hasAttr(const XmlChar *name) const
Check for the existence of a named attribute.
XERCES_XMLCH_T XmlChar
Use the definition from the autoconf header of Xerces:
User abstraction class to manipulate XML elements within a document.
Namespace for the AIDA detector description toolkit.
T attr(const XmlAttr *att) const
Access attribute with implicit return type conversion.