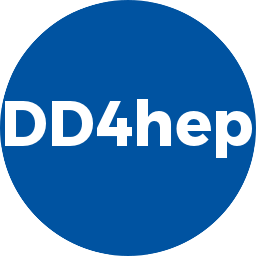 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 namespace DDSegmentation {
29 using std::runtime_error;
30 using std::stringstream;
35 _type =
"TiledLayerSegmentation";
36 _description =
"Cartesian segmentation using optimal tiling depending on the layer dimensions";
56 _type =
"TiledLayerSegmentation";
57 _description =
"Cartesian segmentation using optimal tiling depending on the layer dimensions";
95 "TiledLayerSegmentation::setLayerDimensions: inconsistent size of layer parameter vectors.");
114 "TiledLayerSegmentation::layerDimensions: inconsistent size of layer parameter vectors.");
118 stringstream message;
119 message <<
"TiledLayerSegmentation::layerDimensions: invalid layer index " << layerIndex;
120 throw runtime_error(message.str());
137 return Vector3D(localX, localY, 0.);
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
static double calculateOffset(double cellSize, double totalSize)
helper method to calculate offset of bin 0 based on the total size
Simple container for a physics vector.
double _gridSizeY
default grid size in X
std::string _identifierY
encoding field used for X
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
std::vector< double > dimensions(const Handle< TGeoShape > &solid)
Access Shape dimension parameters (As in TGeo, but angles in radians rather than degrees)
virtual ~TiledLayerSegmentation()
destructor
FieldID get(CellID bitfield, size_t idx) const
Helper class to store x and y dimensions of a layer.
double layerGridSizeX(int layerIndex) const
access the actual grid size in X for a given layer
void setLayerDimensions(int layerIndex, double x, double y)
set the dimensions of the given layer
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
LayerDimensions layerDimensions(int layerIndex) const
access to the dimensions of the given layer
double layerGridSizeY(int layerIndex) const
access the actual grid size in Y for a given layer
void set(CellID &bitfield, size_t idx, FieldID value) const
std::string _identifierLayer
encoding field used for Y
std::string _identifierX
default grid size in Y
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
std::vector< double > _layerDimensionsX
list of valid layer identifiers
std::string _description
The description of the segmentation.
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
static double calculateOptimalCellSize(double nominalCellSize, double totalSize)
list of layer y dimensions
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
Namespace for the AIDA detector description toolkit.
std::string _type
The segmentation type.
double y() const
Access to y value (required for use with ROOT GenVector)
std::vector< int > _layerIndices
encoding field used for the layer
Base class for all segmentations.
double x() const
Access to x value (required for use with ROOT GenVector)
TiledLayerSegmentation(const std::string &cellEncoding="")
Default constructor passing the encoding string.
std::vector< double > _layerDimensionsY
list of layer x dimensions