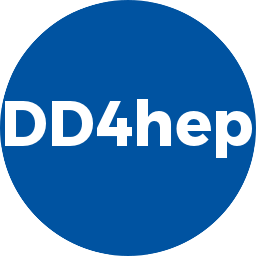 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
27 namespace DDSegmentation {
48 _type =
"MegatileLayerGridXY";
49 _description =
"Cartesian segmentation in the local XY-plane: megatiles, containing integer number of tiles/strips/cells";
87 if ( fabs( cellPosition.
X )>10000e0 || fabs( cellPosition.
Y )>10000e0 ) {
88 printout(WARNING,
"MegatileLayerGridXY",
"crazy cell position: x: %f y: %f ", cellPosition.
X, cellPosition.
Y);
89 printout(WARNING,
"MegatileLayerGridXY",
"layer, wafer, cellx,y indices: %d %d %d %d",
90 layerIndex, waferIndex, cellIndexX, cellIndexY);
91 assert(0 &&
"crazy cell position?");
112 double localX = localPosition.
X;
113 double localY = localPosition.
Y;
138 double sizex,
double sizey,
139 double offsetx,
double offsety,
140 unsigned int ncellsx,
unsigned int ncellsy ) {
142 std::pair <int, int> tileid(layer, tile);
156 std::pair < unsigned int, unsigned int > tileid(layerIndex, waferIndex);
167 assert ( layerIndex<
_nCellsX.size() &&
"MegatileLayerGridXY ERROR: too high layer index?" );
185 return {xsize, ysize};
void setSpecialMegaTile(unsigned int layer, unsigned int tile, double sizex, double sizey, double offsetx, double offsety, unsigned int ncellsx, unsigned int ncellsy)
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
FieldID get(CellID bitfield, size_t idx) const
std::vector< int > _nCellsY
std::string _identifierWafer
encoding field used for the wafer
virtual ~MegatileLayerGridXY()
destructor
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
std::string _identifierModule
std::string _identifierLayer
encoding field used for the layer
MegatileLayerGridXY(const std::string &cellEncoding="")
Default constructor passing the encoding string.
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
void set(CellID &bitfield, size_t idx, FieldID value) const
std::string _xId
the field name used for X
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
std::string _description
The description of the segmentation.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
void getSegInfo(unsigned int layerIndex, unsigned int waferIndex) const
Namespace for the AIDA detector description toolkit.
std::string _type
The segmentation type.
std::vector< int > _nCellsX
std::string _yId
the field name used for Y
std::map< std::pair< unsigned int, unsigned int >, segInfo > specialMegaTiles_layerWafer
Segmentation base class describing cartesian grid segmentation.