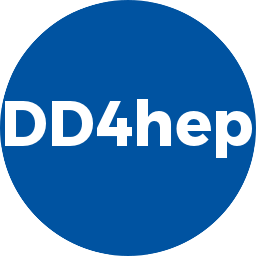 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #ifndef DDG4_GEANT4PARTICLEHANDLER_H
25 #define DDG4_GEANT4PARTICLEHANDLER_H
36 class G4SteppingManager;
77 #if defined(__CINT__) || defined(__MAKECINT__) || defined(G__DICTIONARY)
153 virtual void step(
const G4Step*
step, G4SteppingManager* mgr);
159 virtual void begin(
const G4Track* track);
161 virtual void end(
const G4Track* track);
164 virtual void mark(
const G4Track* track)
override;
166 virtual void mark(
const G4Track* track,
int reason)
override;
170 virtual void mark(
const G4Step*
step,
int reason)
override;
179 #endif // DDG4_GEANT4PARTICLEHANDLER_H
virtual void endEvent(const G4Event *event)
Post-event action callback.
bool m_keepAll
Property: Flag to keep all particles generated.
Geant4ParticleHandler()
No default constructor.
Data structure to map primaries to particles.
Geant4ParticleMap::Particle Particle
Geant4Action to collect the MC particle information.
int recombineParents()
Recombine particles and associate the to parents with cleanup.
Geant4PrimaryMap * m_primaryMap
Primary map.
bool m_printEndTracking
Property: Steer printout at tracking action end.
Processes m_processNames
Property: All the processes of which the decay products will be explicitly stored.
Geant4ParticleHandler & operator=(const Geant4ParticleHandler &c)
No assignment operator.
void checkConsistency() const
Check the record consistency.
virtual void begin(const G4Track *track)
Pre-track action callback.
virtual void mark(const G4Step *step, int reason) override
Store a track produced in a step to be kept for later MC truth analysis.
std::map< int, int > TrackEquivalents
virtual void step(const G4Step *step, G4SteppingManager *mgr)
User stepping callback.
virtual ~Geant4ParticleHandler()
Default destructor.
TrackEquivalents m_equivalentTracks
Map associating the G4Track identifiers with identifiers of existing MCParticles.
std::vector< std::string > Processes
ParticleMap m_suspendedPM
Map with stored MC Particles that were suspended by the stepping action.
void dumpMap(const char *tag) const
Debugging: Dump Geant4 particle map.
Geant4ParticleHandler user extension action called by the particle handler.
void rebaseSimulatedTracks(int base)
Rebase the simulated tracks, so that they fit to the generator particles.
Geant4ParticleMap::TrackEquivalents TrackEquivalents
std::map< int, Particle * > ParticleMap
Default Interface class to handle monte carlo truth records.
virtual void mark(const G4Step *step) override
Mark a Geant4 track of the step to be kept for later MC truth analysis. Default flag: CREATED_HIT.
Concrete implementation of the Geant4 generator action base class.
double m_minDistToParentVertex
Property: Minimal distance after which the vertexIsNotEndpointOfParent flag is set.
bool adopt(Geant4Action *action)
Adopt the user particle handler.
Default base class for all Geant 4 actions and derivates thereof.
static bool defaultKeepParticle(Particle &particle)
Default callback to be answered if the particle should be kept if NO user handler is installed.
int m_globalParticleID
Global particle identifier. Obtained at the begin of the event.
ParticleMap m_particleMap
Map with stored MC Particles.
virtual void operator()(G4Event *event) override
Event generation action callback.
void setVertexEndpointBit()
set the endpointIsNotVertexOfParentFlag at the end of the event
virtual void mark(const G4Track *track, int reason) override
Store a track.
Geant4UserParticleHandler * m_userHandler
User action pointer.
virtual void mark(const G4Track *track) override
Mark a Geant4 track to be kept for later MC truth analysis. Default flag: CREATED_HIT.
bool m_ownsParticles
Property: Flag if the handler is executed in standalone mode and hence must manage particles.
bool m_printStartTracking
Property: Steer printout at tracking action begin.
void clear()
Clear particle maps.
Namespace for the AIDA detector description toolkit.
Particle m_currTrack
Local buffer about the 'current' G4Track.
double m_kinEnergyCut
Property: Energy cut below which particles are not collected, but assigned to the parent.
virtual void end(const G4Track *track)
Post-track action callback.
Data structure to store the MC particle information.
Geant4ParticleMap::ParticleMap ParticleMap
virtual void beginEvent(const G4Event *event)
Pre-event action callback.
Generic context to extend user, run and event information.
Geant4ParticleHandler(Geant4Context *context, const std::string &nam)
Standard constructor.
Geant4Context * context() const
Access the context.