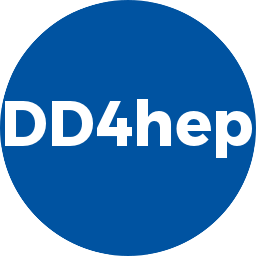 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #include <G4PrimaryVertex.hh>
27 #include <G4PrimaryParticle.hh>
28 #include <G4VPrimaryGenerator.hh>
58 except(
"Geant4GeneratorWrapper: FATAL Failed to create G4VPrimaryGenerator of type %s.",
70 std::set<G4PrimaryVertex*> primaries;
73 generator()->GeneratePrimaryVertex(event);
76 for(G4PrimaryVertex*
v=event->GetPrimaryVertex();
v;
v=
v->GetNext())
Geant4Vertex * createPrimary(const G4PrimaryVertex *g4)
Create a vertex object from its G4 counterpart.
Class modelling a complete primary event with multiple interactions.
Data structure to map primaries to particles.
Geant4GeneratorWrapper(Geant4Context *context, const std::string &nam)
Standard constructor.
Class modelling a single interaction with multiple primary vertices and particles.
static void increment(T *)
Increment count according to type information.
int m_mask
Property: interaction identifier mask. name: "Mask".
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
virtual ~Geant4GeneratorWrapper()
Default destructor.
G4VPrimaryGenerator * m_generator
Reference to the implementation instance.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
Concrete implementation of the Geant4 generator action base class.
virtual void operator()(G4Event *event) override
Event generation action callback.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
G4VPrimaryGenerator * generator()
Access the G4VPrimaryGenerator instance.
std::string m_generatorType
Property: Type name of the implementation instance. name: "Uses".
void add(int id, Geant4PrimaryInteraction *interaction)
Add a new interaction object to the event.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.