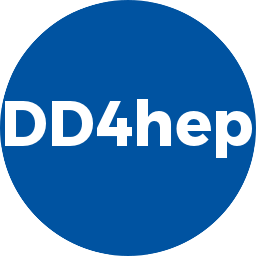 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
17 #include <DD4hep/detail/Handle.inl>
28 void calculate_combined_field(std::vector<CartesianField>&
v,
const Position& pos,
double* field) {
29 for (
const auto& i :
v ) i.value(pos, field);
57 return data<Object>()->properties;
62 double fld[3] = {0e0, 0e0, 0e0};
63 double position[3] = {pos.X(), pos.Y(), pos.Z()};
64 data<Object>()->fieldComponents(position, fld);
65 field =
Direction(fld[0], fld[1], fld[2]);
70 double position[3] = {pos.X(), pos.Y(), pos.Z()};
71 data<Object>()->fieldComponents(position, field);
76 data<Object>()->fieldComponents(pos, field);
94 assign(obj, nam,
"overlay_field");
100 return data<Object>()->properties;
105 int field = data<Object>()->field_type;
112 Object* o = data<Object>();
119 v.emplace_back(field);
125 v.emplace_back(field);
129 if ( isMag || isEle ) {
132 except(
"OverlayedField",
"add: Attempt to add an unknown field type.");
134 except(
"OverlayedField",
"add: Attempt to add an invalid object.");
136 except(
"OverlayedField",
"add: Attempt to add an invalid field.");
142 field[0] = field[1] = field[2] = 0.0;
143 auto* obj = data<Object>();
148 calculate_combined_field(obj->magnetic_components, pos, field);
151 except(
"OverlayedField",
"add: Attempt to add an invalid field.");
156 field[0] = field[1] = field[2] = 0.;
157 calculate_combined_field(data<Object>()->electric_components, pos, field);
162 field[0] = field[1] = field[2] = 0.;
163 calculate_combined_field(data<Object>()->magnetic_components, pos, field);
168 Object* o = data<Object>();
169 field[0] = field[1] = field[2] = 0.;
void combinedElectric(const Position &pos, double *field) const
Returns the 3 electric field components (x, y, z) if many components are present.
bool changesEnergy() const
Does the field change the energy of charged particles?
NamedObject Object
Extern accessible definition of the contained element type.
bool isValid() const
Check the validity of the object held by the handle.
Internal data class shared by all handles of a given type.
void add(CartesianField field)
Add a new field component.
static void increment(T *)
Increment count according to type information.
Properties & properties() const
Access to properties container.
virtual ~Object()
Default destructor.
void combinedMagnetic(const Position &pos, double *field) const
Returns the 3 magnetic field components (x, y, z) if many components are present.
OverlayedField::Object OverlayedFieldObject
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
static void decrement(T *)
Decrement count according to type information.
std::map< std::string, std::map< std::string, std::string > > Properties
Object()
Default constructor.
int fieldType() const
Access the field type.
std::vector< CartesianField > electric_components
NamedObject * m_element
Single and only data member: Reference to the actual element.
const char * GetTitle() const
Get name (used by Handle)
Properties & properties() const
Access to properties container.
Internal data class shared by all handles.
void value(const Position &pos, Direction &field) const
Returns the 3 field components (x, y, z).
ROOT::Math::XYZVector Position
int field_type
Field type.
Object()
Default constructor.
Namespace for the AIDA detector description toolkit.
OverlayedField()=default
Default constructor.
std::vector< CartesianField > magnetic_components
bool changesEnergy() const
Does the field change the energy of charged particles?
CartesianField::Object CartesianFieldObject
void magneticField(const Position &pos, double *field) const
Returns the 3 magnetic field components (x, y, z).
std::map< std::string, PropertyValues > Properties
Internal data class shared by all handles of a given type.
void electromagneticField(const Position &pos, double *field) const
Returns the 3 electric (val[0]-val[2]) and magnetic field components (val[3]-val[5]).
const char * type() const
Access the field type (string)
DD4HEP_INSTANTIATE_HANDLE(CartesianFieldObject)
virtual ~Object()
Default destructor.
Base class describing any field with 3D cartesian vectors for the field strength.