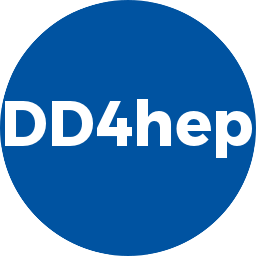 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
36 size_t num_conditions = 0;
37 for(
auto type : types ) {
43 cp.second->select_all(rc);
45 conditions.emplace_back(c);
46 num_conditions += rc.size();
51 return num_conditions;
63 size_t num_conditions = 0;
64 for(
auto type : types ) {
70 cp.second->select_all(rc);
72 conditions.emplace(c->hash,c);
73 num_conditions += rc.size();
78 return num_conditions;
std::vector< Condition > RangeConditions
Elements elements
Container of IOV dependent conditions pools.
const std::vector< const IOVType * > iovTypesUsed() const
Access the used/registered IOV types.
ConditionsIOVPool * iovPool(const IOVType &type) const
Access conditions multi IOV pool by iov type.
static ConditionsManager from(T &host)
Static accessor if installed as an extension.
Namespace for implementation details of the AIDA detector description toolkit.
static size_t collectAllConditions(Detector &description, RangeConditions &conditions)
Select all condition from the conditions manager registered at the Detector object.
Manager class for condition handles.
The main interface to the dd4hep detector description package.
Pool of conditions satisfying one IOV type (epoch, run, fill, etc)