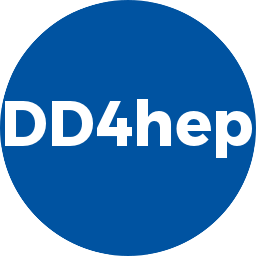 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
23 #include <TEveTrans.h>
24 #include <TEveScene.h>
25 #include <TGLViewer.h>
26 #include <TGLWidget.h>
27 #include <TEveCaloLegoOverlay.h>
28 #include <TEveLegoEventHandler.h>
42 InstanceCount::increment(
this);
46 CaloLego::~CaloLego() {
60 DisplayConfiguration::Configurations::const_iterator j = config.
subdetectors.begin();
64 ctx.
eveHist =
new TEveCaloDataHist();
66 const char* n = (*j).name.c_str();
70 for(
int isl = 0; isl<calo.
eveHist->GetNSlices(); ++isl) {
71 int nslice = ctx.
eveHist->GetNSlices();
72 TH2F* h =
new TH2F(*calo.
eveHist->GetHist(isl));
76 legend_y += a->GetTextSize();
80 TEveCaloLego* lego =
new TEveCaloLego(ctx.
eveHist);
87 lego->InitMainTrans();
88 lego->RefMainTrans().SetScale(TMath::TwoPi(), TMath::TwoPi(), TMath::Pi());
91 TGLViewer* glv =
viewer()->GetGLViewer();
92 TEveCaloLegoOverlay* overlay =
new TEveCaloLegoOverlay();
93 glv->AddOverlayElement(overlay);
94 overlay->SetCaloLego(lego);
97 glv->SetEventHandler(
new TEveLegoEventHandler(glv->GetGLWidget(), glv, lego));
98 glv->SetCurrentCamera(TGLViewer::kCameraOrthoXOY);
99 ctx.
eveHist->GetEtaBins()->SetTitleFont(120);
100 ctx.
eveHist->GetEtaBins()->SetTitle(
"h");
101 ctx.
eveHist->GetPhiBins()->SetTitleFont(120);
102 ctx.
eveHist->GetPhiBins()->SetTitle(
"f");
109 DisplayConfiguration::Configurations::const_iterator j = config.
subdetectors.begin();
110 printout(INFO,
"CaloLego",
"+++ Import geometry topics for view %s.",
name().c_str());
112 const char* n = (*j).name.c_str();
115 for(
int isl = 0; isl<ctx.
eveHist->GetNSlices(); ++isl) {
116 TH2F* global = ctx.
eveHist->GetHist(isl);
The main class of the DDEve display.
virtual void ConfigureGeometry(const DisplayConfiguration::ViewConfig &config) override
Configure a single geometry view.
#define DECLARE_VIEW_FACTORY(x)
static float DefaultTextSize()
Default text size.
virtual void ConfigureEvent(const DisplayConfiguration::ViewConfig &config) override
Configure a single event scene view.
union dd4hep::DisplayConfiguration::Config::Values data
virtual View & Map(TEveWindow *slot)
Map the view view to the slot.
Lego plot for calorimeter energy deposits.
Configurations subdetectors
void ImportGeoTopics(const std::string &title) override
Call to import geometry topics.
Display::CalodataContext m_data
ClassImp(CaloLego) CaloLego
Initializing constructor.
const std::string & name() const
Access to the view name/title.
CalodataContext & GetCaloHistogram(const std::string &name)
Access to calo data histograms by name as defined in the configuration.
TEveCaloDataHist * eveHist
virtual TEveElementList * AddToGlobalItems(const std::string &nam)
Add the view to the global list of eve objects.
View TEveWindowSlot * slot
static void decrement(T *)
Decrement count according to type information.
virtual View & CreateGeoScene()
Create the geometry scene.
static float DefaultMargin()
Default margin for placement in bottom left corner.
DisplayConfiguration::Config config
TEveViewer * viewer() const
Access to the Eve viewer.
Class to add annotations to eve viewers.
Configuration class for 3D calorimeter data display.
Namespace for the AIDA detector description toolkit.
class View View.h DDEve/View.h
Calorimeter data context for the DDEve event display.
virtual View & Build(TEveWindow *slot) override
Build the projection view and map it to the given slot.
CaloLego(Display *eve, const std::string &name)
Initializing constructor.
virtual void ImportGeo(const std::string &topic, TEveElement *element)
Call to import geometry elements into topics.