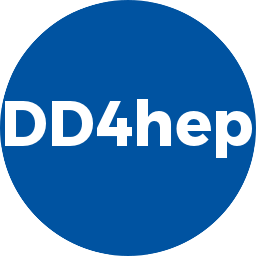 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
45 class Path :
public std::string {
58 template <
class Iter>
Path(Iter _begin,Iter _end) {
59 if ( _begin != _end ) {
60 std::string str(_begin, _end);
61 this->std::string::operator=(std::move(str));
68 this->std::string::operator=(
copy);
73 this->std::string::operator=(
copy);
78 this->std::string::operator=(std::move(
copy));
83 this->std::string::operator=(std::move(
copy));
104 const std::string&
native()
const {
return *
this; }
106 const char*
string_data()
const {
return this->std::string::c_str(); }
119 #endif // DD4HEP_PATH_H
Path & operator/=(const std::string ©)
Append operation.
Path(const Path ©)
Copy constructor.
Path & append(const std::string ©)
Append operation.
static const Path & dot_dot_path()
Path representing "..".
Path & remove_filename()
Manipulator: remove the file name part. Leaves the parent path.
Path(Path &©)
Move constructor.
const std::string & native() const
String representation of thre Path object.
Path(const std::string ©)
Initializing constructor.
Path & operator=(Path &©)
Move assignment operator from Path object.
Path filename() const
The file name of the path.
Path & operator=(const Path ©)
Assignment operator from Path object.
~Path()
Default destructor.
Path & operator/=(const Path ©)
Append operation.
Path file_path() const
The full file path of the object.
Path normalize() const
Normalize path name.
size_t parent_path_end() const
Index of the parent's path end.
Path & operator=(std::string &©)
Assignment operator from string object.
Path(Iter _begin, Iter _end)
Assigning constructor.
const char * string_data() const
String representation of thre Path object.
Path & operator=(const std::string ©)
Assignment operator from string object.
Namespace for the AIDA detector description toolkit.
Path(std::string &©)
Initializing constructor.
Path()
Default constructor.
static const Path & dot_path()
Path representing ".".
Path parent_path() const
Parent's path.