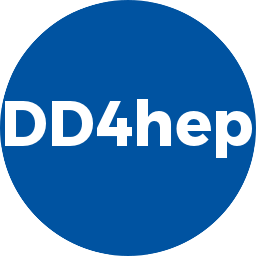 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef XML_LAYERING_H
15 #define XML_LAYERING_H
38 LayerSlice(
bool sens,
double thick,
const std::string& mat);
47 : _sensitive(sens), _thickness(thick), _material(mat) {
101 const std::vector<Layer*>&
layers()
const {
163 #endif // XML_LAYERING_H
double totalThickness() const
double absorberThicknessInLayer(xml::Element e) const
Layering()=default
Default constructor.
Layer & operator=(const Layer ©)=default
Assignment operator.
LayerStack()=default
Default constructor.
const Layer * layer(size_t which) const
void fromCompact(Layering &layering) const
Invoke converter.
double thicknessWithPreOffset() const
Class to describe a layering stack.
XML converter for layering objects.
LayerSlice(const LayerSlice ©)=default
Copy constructor.
LayerSlice(bool sens, double thick, const std::string &mat)
Initializing constructor.
Class to describe one layer in a layering stack.
Layer()=default
Default constructor.
double totalThickness() const
std::vector< Layer * > & layers()
void sensitivePositionsInLayer(xml::Element e, std::vector< double > &sens_pos) const
void add(const LayerSlice &slice)
Namespace for the AIDA detector description toolkit supporting XML utilities.
double sectionThickness(size_t is, size_t ie) const
LayerStack(const LayerStack ©)=default
Copy constructor.
Class to convert a layering object from the compact notation.
Class to describe the slice of one layer in a layering stack.
~LayerStack()=default
Default destructor.
User abstraction class to manipulate XML elements within a document.
Namespace for the AIDA detector description toolkit.
LayerStack & operator=(const LayerStack ©)=default
Assignment operator.
const std::vector< Layer * > & layers() const
double singleLayerThickness(xml::Element e) const
std::vector< Layer * > & layers()
std::vector< LayerSlice > _slices
LayerSlice & operator=(const LayerSlice ©)=default
Assignment operator.
std::vector< Layer * > _layers
LayeringCnv(Element e)
Initializing constructor.
~Layering()
Default destructor.
Layer(const Layer ©)=default
Copy constructor.