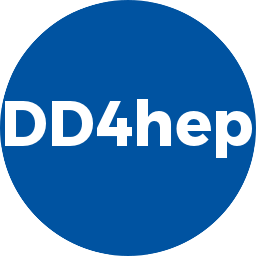 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <G4ParticleDefinition.hh>
25 #include <CLHEP/Units/SystemOfUnits.h>
30 bool user_limit_debug =
false;
35 bool tmp = user_limit_debug;
36 user_limit_debug = value;
42 auto* def = track.GetParticleDefinition();
46 if ( user_limit_debug ) {
47 dd4hep::printout(dd4hep::INFO,
"Geant4UserLimits",
"Apply explicit limit %f to track: %s",
48 def->GetParticleName().c_str());
53 if ( user_limit_debug ) {
54 dd4hep::printout(dd4hep::INFO,
"Geant4UserLimits",
"Apply default limit %f to track: %s",
55 def->GetParticleName().c_str());
62 if ( particles ==
"*" || particles ==
".(.*)" ) {
67 for(
auto* d : defs ) {
68 particleLimits[d] = val;
87 const auto& lim = limitset.
limits();
95 for(
const Limit& l : lim) {
96 if (l.name ==
"step_length_max")
98 else if (l.name ==
"track_length_max")
100 else if (l.name ==
"time_max")
101 maxTime.
set(l.particles, l.value*CLHEP::ns/dd4hep::ns);
102 else if (l.name ==
"ekin_min")
103 minEKine.
set(l.particles, l.value*CLHEP::MeV/dd4hep::MeV);
104 else if (l.name ==
"range_min")
107 except(
"Geant4UserLimits",
"Unknown Geant4 user limit: %s ", l.toString().c_str());
113 dd4hep::notImplemented(std::string(__PRETTY_FUNCTION__)+
" May not be called!");
117 dd4hep::notImplemented(std::string(__PRETTY_FUNCTION__)+
" May not be called!");
121 dd4hep::notImplemented(std::string(__PRETTY_FUNCTION__)+
" May not be called!");
125 dd4hep::notImplemented(std::string(__PRETTY_FUNCTION__)+
" May not be called!");
129 dd4hep::notImplemented(std::string(__PRETTY_FUNCTION__)+
" May not be called!");
Handler minRange
Handler map for MinRange limit.
const std::set< Limit > & limits() const
Accessor to limits container.
Small object describing a limit structure acting on a particle type.
static std::vector< G4ParticleDefinition * > g4DefinitionsRegEx(const std::string &expression)
Access Geant4 particle definitions by regular expression.
static void increment(T *)
Increment count according to type information.
Geant4UserLimits(LimitSet limitset)
Initializing Constructor.
Handler minEKine
Handler map for MinEKine limit.
Handler maxTrackLength
Handler map for MaxTrackLength limit.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual void SetUserMinRange(G4double urangMin) override
virtual ~Geant4UserLimits()
Standard destructor.
LimitSet limits
Handle to the limitset to be applied.
Handler maxTime
Handler map for MaxTime limit.
virtual void SetUserMaxTime(G4double utimeMax) override
Handle class describing a set of limits as they are used for simulation.
virtual void SetUserMaxTrackLength(G4double utrakMax) override
static void decrement(T *)
Decrement count according to type information.
std::map< const G4ParticleDefinition *, double > particleLimits
Handler particle ids for the limit (pdgID)
virtual void SetMaxAllowedStep(G4double ustepMax) override
Setters may not be called!
Handler maxStepLength
Handler map for MaxStepLength limit.
double defaultValue
Default value (either from base class or value if Limit.particles='*')
virtual void SetUserMinEkine(G4double uekinMin) override
virtual void update(LimitSet limitset)
Update the object.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
double value(const G4Track &track) const
Access value according to track.
static bool enable_debug(bool value)
Allow for debugging user limits (very verbose)
void set(const std::string &particles, double val)
Set the handler value(s)